namespace
PrimitivesPrimitive library.
Basic primitives for testing purposes.
This library is built if MAGNUM_WITH_PRIMITIVES
is enabled when building Magnum. To use this library with CMake, request the Primitives
component of the Magnum
package and link to the Magnum::Primitives
target:
find_package(Magnum REQUIRED Primitives) # ... target_link_libraries(your-app PRIVATE Magnum::Primitives)
See Downloading and building and Usage with CMake for more information.
Enums
- enum class CapsuleFlag: UnsignedByte { TextureCoordinates = 1 << 0, Tangents = 1 << 1 new in 2020.06 } new in 2020.06
- Capsule flag.
- enum class CapsuleTextureCoords: UnsignedByte { DontGenerate, Generate } deprecated in 2020.06
- Whether to generate capsule texture coordinates.
- enum class Circle2DFlag: UnsignedByte { TextureCoordinates = 1 << 0 } new in 2020.06
- 2D circle flag
- enum class CircleTextureCoords: UnsignedByte { DontGenerate, Generate } deprecated in 2020.06
- Whether to generate circle texture coordinates.
- enum class Circle3DFlag: UnsignedByte { TextureCoordinates = 1 << 0, Tangents = 1 << 1 new in 2020.06 } new in 2020.06
- 3D circle flag
- enum class ConeFlag: UnsignedByte { TextureCoordinates = 1 << 0 new in 2020.06, GenerateTextureCoords = TextureCoordinates deprecated in 2020.06, Tangents = 1 << 1 new in 2020.06, CapEnd = 1 << 2 }
- Cone flag.
- enum class CylinderFlag: UnsignedByte { TextureCoordinates = 1 << 0 new in 2020.06, GenerateTextureCoords = TextureCoordinates deprecated in 2020.06, Tangents = 1 << 1 new in 2020.06, CapEnds = 1 << 2 }
- Cylinder flag.
- enum class GridFlag: UnsignedByte { TextureCoordinates = 1 << 0 new in 2020.06, GenerateTextureCoords = TextureCoordinates deprecated in 2020.06, Normals = 1 << 1, GenerateNormals = Normals deprecated in 2020.06, Tangents = 1 << 2 new in 2020.06 }
- Grid flag.
- enum class PlaneFlag: UnsignedByte { TextureCoordinates = 1 << 0, Tangents = 1 << 1 new in 2020.06 } new in 2020.06
- Plane flag.
- enum class PlaneTextureCoords: UnsignedByte { DontGenerate, Generate } deprecated in 2020.06
- Whether to generate plane texture coordinates.
- enum class SquareFlag: UnsignedByte { TextureCoordinates = 1 << 0 } new in 2020.06
- Square flag.
- enum class SquareTextureCoords: UnsignedByte { DontGenerate, Generate } deprecated in 2020.06
- Whether to generate square texture coordinates.
- enum class UVSphereFlag: UnsignedByte { TextureCoordinates = 1 << 0, Tangents = 1 << 1 new in 2020.06 } new in 2020.06
- UV sphere flag.
- enum class UVSphereTextureCoords: UnsignedByte { DontGenerate, Generate } deprecated in 2020.06
- Whether to generate UV sphere texture coordinates.
Typedefs
-
using CapsuleFlags = Containers::
EnumSet<CapsuleFlag> new in 2020.06 - Capsule flags.
-
using Circle2DFlags = Containers::
EnumSet<Circle2DFlag> new in 2020.06 - 2D circle flags
-
using Circle3DFlags = Containers::
EnumSet<Circle3DFlag> new in 2020.06 - 3D circle flags
-
using ConeFlags = Containers::
EnumSet<ConeFlag> - Cone flags.
-
using CylinderFlags = Containers::
EnumSet<CylinderFlag> - Cylinder flags.
-
using GridFlags = Containers::
EnumSet<GridFlag> - Grid flags.
-
using PlaneFlags = Containers::
EnumSet<PlaneFlag> new in 2020.06 - Plane flags.
-
using SquareFlags = Containers::
EnumSet<SquareFlag> new in 2020.06 - Square flags.
-
using UVSphereFlags = Containers::
EnumSet<UVSphereFlag> new in 2020.06 - UV sphere flags.
Functions
-
auto axis2D() -> Trade::
MeshData - 2D axis
-
auto axis3D() -> Trade::
MeshData - 3D axis
-
auto capsule2DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
Float halfLength) -> Trade::
MeshData - Wireframe 2D capsule.
-
auto capsule3DSolid(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength,
CapsuleFlags flags = {}) -> Trade::
MeshData new in 2020.06 - Solid 3D capsule.
-
auto capsule3DSolid(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength,
CapsuleTextureCoords textureCoords) -> Trade::
MeshData deprecated in 2020.06 - Solid 3D capsule.
-
auto capsule3DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength) -> Trade::
MeshData - Wireframe 3D capsule.
-
auto circle2DSolid(UnsignedInt segments,
Circle2DFlags flags = {}) -> Trade::
MeshData new in 2020.06 - Solid 2D circle.
-
auto circle2DSolid(UnsignedInt segments,
CircleTextureCoords textureCoords) -> Trade::
MeshData deprecated in 2020.06 - Solid 2D circle.
-
auto circle2DWireframe(UnsignedInt segments) -> Trade::
MeshData - Wireframe 2D circle.
-
auto circle3DSolid(UnsignedInt segments,
Circle3DFlags flags = {}) -> Trade::
MeshData new in 2020.06 - Solid 3D circle.
-
auto circle3DSolid(UnsignedInt segments,
CircleTextureCoords textureCoords) -> Trade::
MeshData deprecated in 2020.06 - Solid 3D circle.
-
auto circle3DWireframe(UnsignedInt segments) -> Trade::
MeshData - Wireframe 3D circle.
-
auto coneSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
ConeFlags flags = {}) -> Trade::
MeshData - Solid 3D cone.
-
auto coneWireframe(UnsignedInt segments,
Float halfLength) -> Trade::
MeshData - Wireframe 3D cone.
-
auto crosshair2D() -> Trade::
MeshData - 2D crosshair
-
auto crosshair3D() -> Trade::
MeshData - 3D crosshair
-
auto cubeSolid() -> Trade::
MeshData - Solid 3D cube.
-
auto cubeSolidStrip() -> Trade::
MeshData - Solid 3D cube as a single strip.
-
auto cubeWireframe() -> Trade::
MeshData - Wireframe 3D cube.
-
auto cylinderSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
CylinderFlags flags = {}) -> Trade::
MeshData - Solid 3D cylinder.
-
auto cylinderWireframe(UnsignedInt rings,
UnsignedInt segments,
Float halfLength) -> Trade::
MeshData - Wireframe 3D cylinder.
-
auto gradient2D(const Vector2& a,
const Color4& colorA,
const Vector2& b,
const Color4& colorB) -> Trade::
MeshData - 2D square with a gradient
-
auto gradient2DHorizontal(const Color4& colorLeft,
const Color4& colorRight) -> Trade::
MeshData - 2D square with a horizontal gradient
-
auto gradient2DVertical(const Color4& colorBottom,
const Color4& colorTop) -> Trade::
MeshData - 2D square with a vertical gradient
-
auto gradient3D(const Vector3& a,
const Color4& colorA,
const Vector3& b,
const Color4& colorB) -> Trade::
MeshData - 3D plane with a gradient
-
auto gradient3DHorizontal(const Color4& colorLeft,
const Color4& colorRight) -> Trade::
MeshData - 3D plane with a horizontal gradient
-
auto gradient3DVertical(const Color4& colorBottom,
const Color4& colorTop) -> Trade::
MeshData - 3D plane with a vertical gradient
-
auto grid3DSolid(const Vector2i& subdivisions,
GridFlags flags = GridFlag::
Normals) -> Trade:: MeshData - Solid 3D grid.
-
auto grid3DWireframe(const Vector2i& subdivisions) -> Trade::
MeshData - Wireframe 3D grid.
-
auto icosphereSolid(UnsignedInt subdivisions) -> Trade::
MeshData - Solid 3D icosphere.
-
auto icosphereWireframe() -> Trade::
MeshData new in 2020.06 - Wireframe 3D icosphere.
-
auto line2D(const Vector2& a,
const Vector2& b) -> Trade::
MeshData - 2D line
-
auto line2D() -> Trade::
MeshData - 2D line in an identity transformation
-
auto line3D(const Vector3& a,
const Vector3& b) -> Trade::
MeshData - 3D line
-
auto line3D() -> Trade::
MeshData - 3D line in an identity transformation
-
auto planeSolid(PlaneFlags flags) -> Trade::
MeshData new in 2020.06 - Solid 3D plane.
-
auto planeSolid() -> Trade::
MeshData -
auto planeSolid(PlaneTextureCoords textureCoords) -> Trade::
MeshData deprecated in 2020.06 - Solid 3D plane.
-
auto planeWireframe() -> Trade::
MeshData - Wireframe 3D plane.
-
auto squareSolid(SquareFlags flags = {}) -> Trade::
MeshData new in 2020.06 - Solid 2D square.
-
auto squareSolid(SquareTextureCoords textureCoords) -> Trade::
MeshData deprecated in 2020.06 - Solid 3D plane.
-
auto squareWireframe() -> Trade::
MeshData - Wireframe 2D square.
-
auto uvSphereSolid(UnsignedInt rings,
UnsignedInt segments,
UVSphereFlags flags = {}) -> Trade::
MeshData new in 2020.06 - Solid 3D UV sphere.
-
auto uvSphereSolid(UnsignedInt rings,
UnsignedInt segments,
UVSphereTextureCoords textureCoords) -> Trade::
MeshData deprecated in 2020.06 - Solid 3D UV sphere.
-
auto uvSphereWireframe(UnsignedInt rings,
UnsignedInt segments) -> Trade::
MeshData - Wireframe 3D UV sphere.
Enum documentation
enum class Magnum:: Primitives:: CapsuleFlag: UnsignedByte new in 2020.06
#include <Magnum/Primitives/Capsule.h>
Capsule flag.
Enumerators | |
---|---|
TextureCoordinates |
Generate texture coordinates |
Tangents new in 2020.06 |
Generate four-component tangents. The last component can be used to reconstruct a bitangent as described in the documentation of Trade:: |
enum class Magnum:: Primitives:: CapsuleTextureCoords: UnsignedByte
#include <Magnum/Primitives/Capsule.h>
Whether to generate capsule texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates |
enum class Magnum:: Primitives:: Circle2DFlag: UnsignedByte new in 2020.06
#include <Magnum/Primitives/Circle.h>
2D circle flag
Enumerators | |
---|---|
TextureCoordinates |
Generate texture coordinates |
enum class Magnum:: Primitives:: CircleTextureCoords: UnsignedByte
#include <Magnum/Primitives/Circle.h>
Whether to generate circle texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates |
enum class Magnum:: Primitives:: Circle3DFlag: UnsignedByte new in 2020.06
#include <Magnum/Primitives/Circle.h>
3D circle flag
Enumerators | |
---|---|
TextureCoordinates |
Generate texture coordinates |
Tangents new in 2020.06 |
Generate four-component tangents. The last component can be used to reconstruct a bitangent as described in the documentation of Trade:: |
enum class Magnum:: Primitives:: ConeFlag: UnsignedByte
#include <Magnum/Primitives/Cone.h>
Cone flag.
Enumerators | |
---|---|
TextureCoordinates new in 2020.06 |
Generate texture coordinates |
GenerateTextureCoords |
Generate texture coordinates |
Tangents new in 2020.06 |
Generate four-component tangents. The last component can be used to reconstruct a bitangent as described in the documentation of Trade:: |
CapEnd |
Cap end |
enum class Magnum:: Primitives:: CylinderFlag: UnsignedByte
#include <Magnum/Primitives/Cylinder.h>
Cylinder flag.
Enumerators | |
---|---|
TextureCoordinates new in 2020.06 |
Generate texture coordinates |
GenerateTextureCoords |
Generate texture coordinates |
Tangents new in 2020.06 |
Generate four-component tangents. The last component can be used to reconstruct a bitangent as described in the documentation of Trade:: |
CapEnds |
Cap ends |
enum class Magnum:: Primitives:: GridFlag: UnsignedByte
#include <Magnum/Primitives/Grid.h>
Grid flag.
Enumerators | |
---|---|
TextureCoordinates new in 2020.06 |
Generate texture coordinates with origin in the bottom left corner |
GenerateTextureCoords |
Generate texture coordinates with origin in the bottom left corner |
Normals |
Generate normals in positive Z direction. Disable if you'd be generating your own normals anyway (for example based on a heightmap). |
GenerateNormals |
Generate normals in positive Z direction. |
Tangents new in 2020.06 |
Generate four-component tangents. The last component can be used to reconstruct a bitangent as described in the documentation of Trade:: |
enum class Magnum:: Primitives:: PlaneFlag: UnsignedByte new in 2020.06
#include <Magnum/Primitives/Plane.h>
Plane flag.
Enumerators | |
---|---|
TextureCoordinates |
Generate texture coordinates with origin in bottom left corner |
Tangents new in 2020.06 |
Generate four-component tangents. The last component can be used to reconstruct a bitangent as described in the documentation of Trade:: |
enum class Magnum:: Primitives:: PlaneTextureCoords: UnsignedByte
#include <Magnum/Primitives/Plane.h>
Whether to generate plane texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates with origin in bottom left corner. |
enum class Magnum:: Primitives:: SquareFlag: UnsignedByte new in 2020.06
#include <Magnum/Primitives/Square.h>
Square flag.
Enumerators | |
---|---|
TextureCoordinates |
Generate texture coordinates with origin in bottom left corner |
enum class Magnum:: Primitives:: SquareTextureCoords: UnsignedByte
#include <Magnum/Primitives/Square.h>
Whether to generate square texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates with origin in bottom left corner. |
enum class Magnum:: Primitives:: UVSphereFlag: UnsignedByte new in 2020.06
#include <Magnum/Primitives/UVSphere.h>
UV sphere flag.
Enumerators | |
---|---|
TextureCoordinates |
Generate texture coordinates |
Tangents new in 2020.06 |
Generate four-component tangents. The last component can be used to reconstruct a bitangent as described in the documentation of Trade:: |
enum class Magnum:: Primitives:: UVSphereTextureCoords: UnsignedByte
#include <Magnum/Primitives/UVSphere.h>
Whether to generate UV sphere texture coordinates.
Enumerators | |
---|---|
DontGenerate |
Don't generate texture coordinates |
Generate |
Generate texture coordinates |
Typedef documentation
typedef Containers:: EnumSet<CapsuleFlag> Magnum:: Primitives:: CapsuleFlags new in 2020.06
#include <Magnum/Primitives/Capsule.h>
Capsule flags.
typedef Containers:: EnumSet<Circle2DFlag> Magnum:: Primitives:: Circle2DFlags new in 2020.06
#include <Magnum/Primitives/Circle.h>
2D circle flags
typedef Containers:: EnumSet<Circle3DFlag> Magnum:: Primitives:: Circle3DFlags new in 2020.06
#include <Magnum/Primitives/Circle.h>
3D circle flags
typedef Containers:: EnumSet<ConeFlag> Magnum:: Primitives:: ConeFlags
#include <Magnum/Primitives/Cone.h>
Cone flags.
typedef Containers:: EnumSet<CylinderFlag> Magnum:: Primitives:: CylinderFlags
#include <Magnum/Primitives/Cylinder.h>
Cylinder flags.
typedef Containers:: EnumSet<GridFlag> Magnum:: Primitives:: GridFlags
#include <Magnum/Primitives/Grid.h>
Grid flags.
typedef Containers:: EnumSet<PlaneFlag> Magnum:: Primitives:: PlaneFlags new in 2020.06
#include <Magnum/Primitives/Plane.h>
Plane flags.
typedef Containers:: EnumSet<SquareFlag> Magnum:: Primitives:: SquareFlags new in 2020.06
#include <Magnum/Primitives/Square.h>
Square flags.
typedef Containers:: EnumSet<UVSphereFlag> Magnum:: Primitives:: UVSphereFlags new in 2020.06
#include <Magnum/Primitives/UVSphere.h>
UV sphere flags.
Function documentation
Trade:: MeshData Magnum:: Primitives:: axis2D()
#include <Magnum/Primitives/Axis.h>
2D axis
Two color-coded arrows for visualizing orientation (XY is RG), going from 0.0f
to 1.0f
on the X and Y axis. MeshPrimitive::
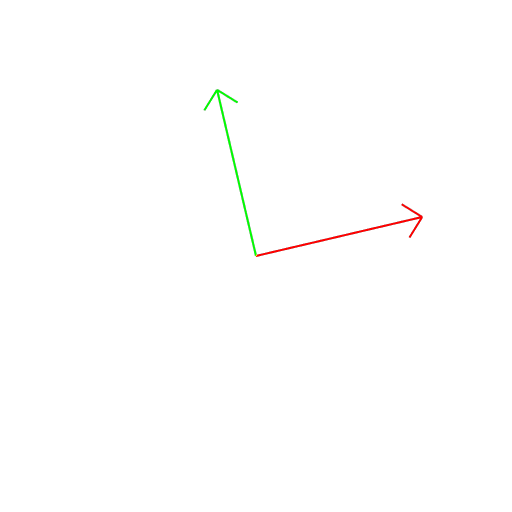
Trade:: MeshData Magnum:: Primitives:: axis3D()
#include <Magnum/Primitives/Axis.h>
3D axis
Three color-coded arrows for visualizing orientation (XYZ is RGB), going from 0.0f
to 1.0f
on the X, Y and Z axis. MeshPrimitive::
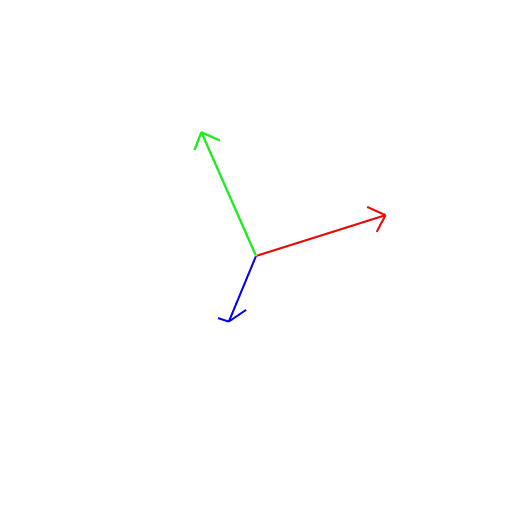
Trade:: MeshData Magnum:: Primitives:: capsule2DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
Float halfLength)
#include <Magnum/Primitives/Capsule.h>
Wireframe 2D capsule.
Parameters | |
---|---|
hemisphereRings | Number of (line) rings for each hemisphere. Must be larger or equal to 1 . |
cylinderRings | Number of (line) rings for cylinder. Must be larger or equal to 1 . |
halfLength | Half the length of cylinder part |
Cylinder of radius 1.0f
along the Y axis, centered at origin, with hemispheres instead of caps. MeshPrimitive::
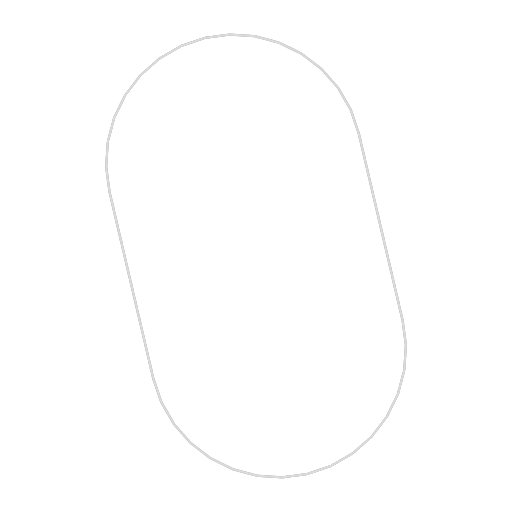
Trade:: MeshData Magnum:: Primitives:: capsule3DSolid(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength,
CapsuleFlags flags = {}) new in 2020.06
#include <Magnum/Primitives/Capsule.h>
Solid 3D capsule.
Parameters | |
---|---|
hemisphereRings | Number of (face) rings for each hemisphere. Must be larger or equal to 1 . |
cylinderRings | Number of (face) rings for cylinder. Must be larger or equal to 1 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
halfLength | Half the length of cylinder part |
flags | Flags |
Cylinder of radius 1.0f
along the Y axis, centered at origin, with hemispheres instead of caps. MeshPrimitive::
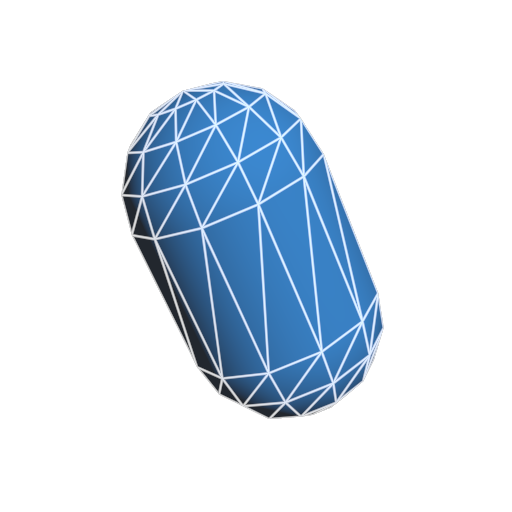
The capsule is by default created with radius set to . In order to get radius , length and preserve correct normals, set halfLength
to and then scale all positions by , for example using MeshTools::
Trade:: MeshData Magnum:: Primitives:: capsule3DSolid(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength,
CapsuleTextureCoords textureCoords)
#include <Magnum/Primitives/Capsule.h>
Solid 3D capsule.
Trade:: MeshData Magnum:: Primitives:: capsule3DWireframe(UnsignedInt hemisphereRings,
UnsignedInt cylinderRings,
UnsignedInt segments,
Float halfLength)
#include <Magnum/Primitives/Capsule.h>
Wireframe 3D capsule.
Parameters | |
---|---|
hemisphereRings | Number of (line) rings for each hemisphere. Must be larger or equal to 1 . |
cylinderRings | Number of (line) rings for cylinder. Must be larger or equal to 1 . |
segments | Number of line segments. Must be larger or equal to 4 and multiple of 4 . |
halfLength | Half the length of cylinder part |
Cylinder of radius 1.0f
along the Y axis, centered at origin, with hemispheres instead of caps. MeshPrimitive::
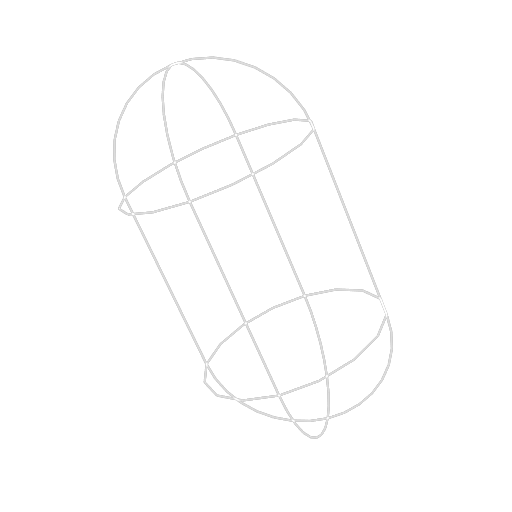
Trade:: MeshData Magnum:: Primitives:: circle2DSolid(UnsignedInt segments,
Circle2DFlags flags = {}) new in 2020.06
#include <Magnum/Primitives/Circle.h>
Solid 2D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
flags | Flags |
Circle with radius 1.0f
, centered at origin. MeshPrimitive::
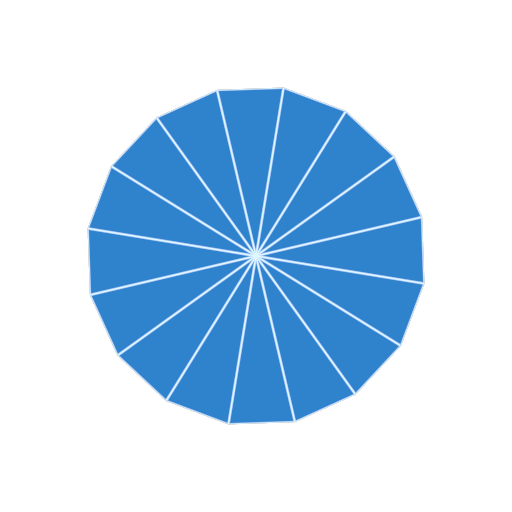
Trade:: MeshData Magnum:: Primitives:: circle2DSolid(UnsignedInt segments,
CircleTextureCoords textureCoords)
#include <Magnum/Primitives/Circle.h>
Solid 2D circle.
Trade:: MeshData Magnum:: Primitives:: circle2DWireframe(UnsignedInt segments)
#include <Magnum/Primitives/Circle.h>
Wireframe 2D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
Circle with radius 1.0f
, centered at origin. Non-indexed MeshPrimitive::
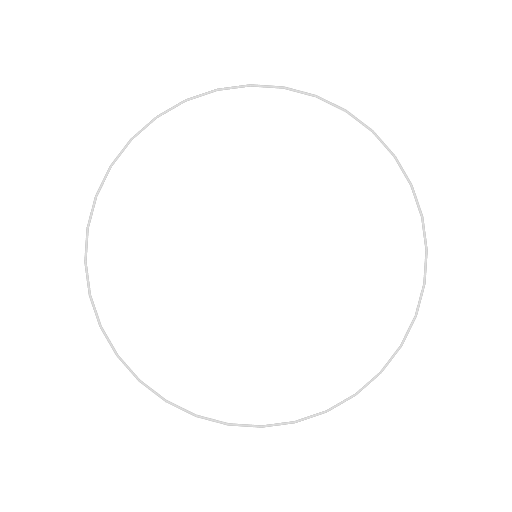
Trade:: MeshData Magnum:: Primitives:: circle3DSolid(UnsignedInt segments,
Circle3DFlags flags = {}) new in 2020.06
#include <Magnum/Primitives/Circle.h>
Solid 3D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
flags | Flags |
Circle on the XY plane with radius 1.0f
, centered at origin. Non-indexed MeshPrimitive::
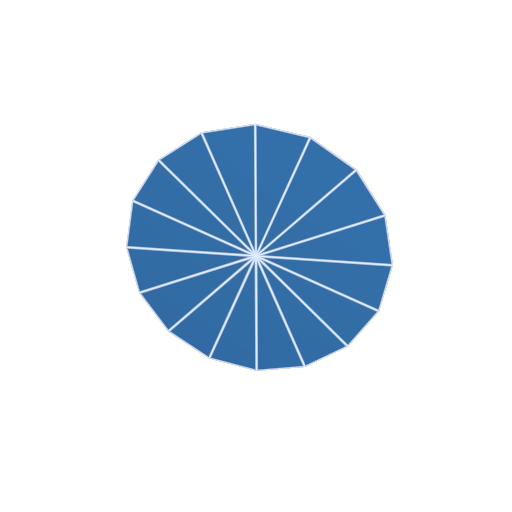
Trade:: MeshData Magnum:: Primitives:: circle3DSolid(UnsignedInt segments,
CircleTextureCoords textureCoords)
#include <Magnum/Primitives/Circle.h>
Solid 3D circle.
Trade:: MeshData Magnum:: Primitives:: circle3DWireframe(UnsignedInt segments)
#include <Magnum/Primitives/Circle.h>
Wireframe 3D circle.
Parameters | |
---|---|
segments | Number of segments. Must be greater or equal to 3 . |
Circle on the XY plane with radius 1.0f
, centered at origin. Non-indexed MeshPrimitive::
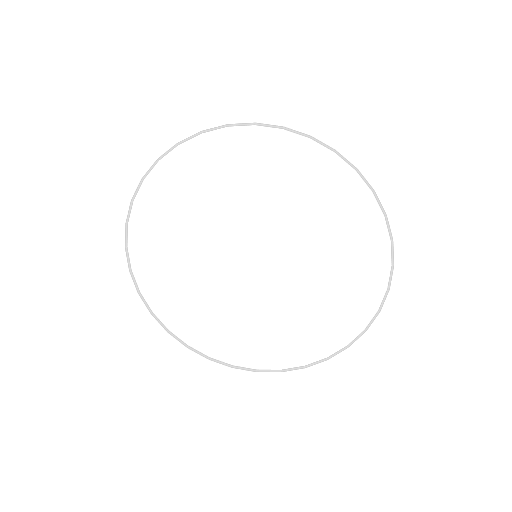
Trade:: MeshData Magnum:: Primitives:: coneSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
ConeFlags flags = {})
#include <Magnum/Primitives/Cone.h>
Solid 3D cone.
Parameters | |
---|---|
rings | Number of (face) rings. Must be larger or equal to 1 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
halfLength | Half the cone length |
flags | Flags |
Cone of radius 1.0f
along the Y axis, centered at origin. MeshPrimitive::segments*2
vertices instead of just one.
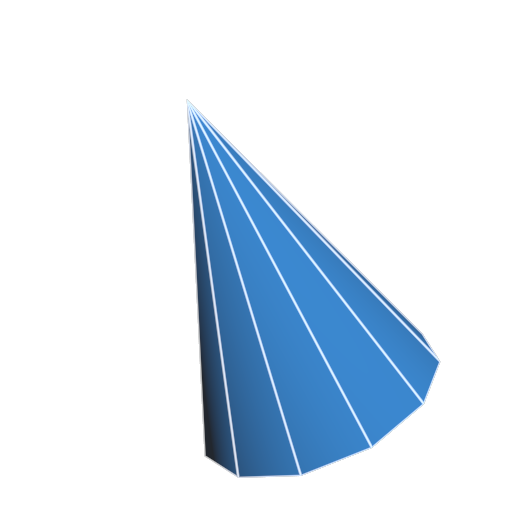
The cone is by default created with radius set to . In order to get radius , length and preserve correct normals, set halfLength
to and then scale all positions by , for example using MeshTools::
Trade:: MeshData Magnum:: Primitives:: coneWireframe(UnsignedInt segments,
Float halfLength)
#include <Magnum/Primitives/Cone.h>
Wireframe 3D cone.
Parameters | |
---|---|
segments | Number of (line) segments. Must be larger or equal to 4 and multiple of 4 . |
halfLength | Half the cone length |
Cone of radius 1.0f
along the Y axis, centered at origin. MeshPrimitive::
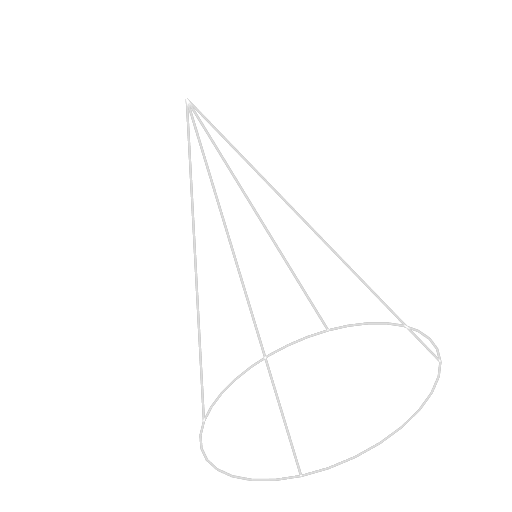
Trade:: MeshData Magnum:: Primitives:: crosshair2D()
#include <Magnum/Primitives/Crosshair.h>
2D crosshair
2x2 crosshair (two crossed lines), centered at origin. Non-indexed MeshPrimitive::
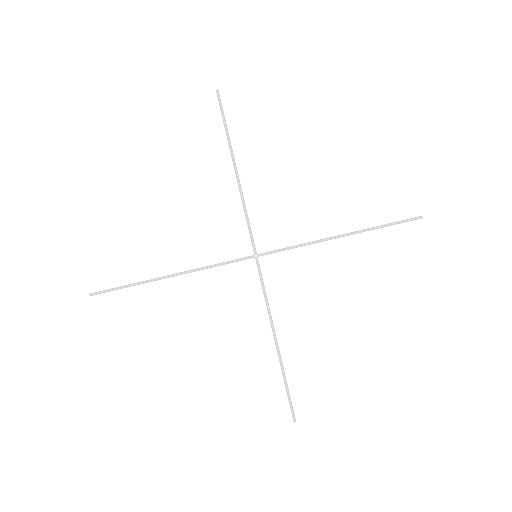
Trade:: MeshData Magnum:: Primitives:: crosshair3D()
#include <Magnum/Primitives/Crosshair.h>
3D crosshair
2x2x2 crosshair (three crossed lines), centered at origin. Non-indexed MeshPrimitive::
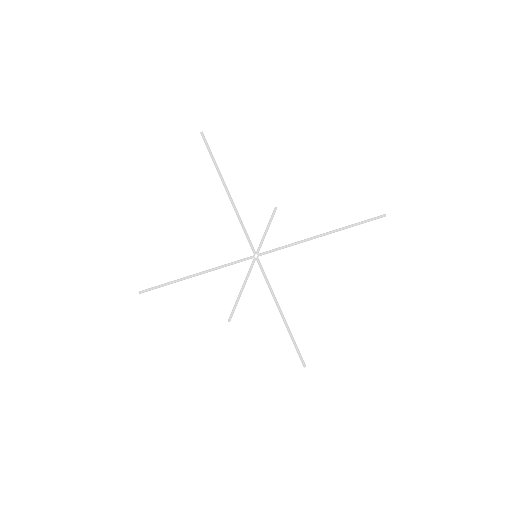
Trade:: MeshData Magnum:: Primitives:: cubeSolid()
#include <Magnum/Primitives/Cube.h>
Solid 3D cube.
2x2x2 cube, centered at origin. MeshPrimitive::
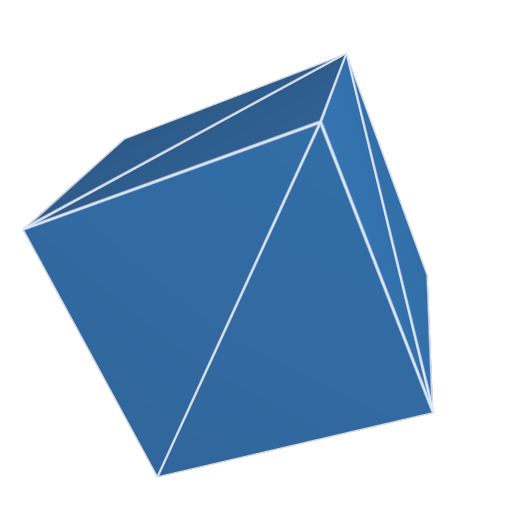
Trade:: MeshData Magnum:: Primitives:: cubeSolidStrip()
#include <Magnum/Primitives/Cube.h>
Solid 3D cube as a single strip.
2x2x2 cube, centered at origin. Non-indexed MeshPrimitive::
Vertex positions of this mesh can be also generated directly in the vertex shader using gl_VertexID
(source, adapted to exactly match the output of this function):
int b = 1 << gl_VertexID; vec3 corner = vec3(mix(-1.0, 1.0, bool(0xd785 & b)), mix(-1.0, 1.0, bool(0x31e3 & b)), mix(-1.0, 1.0, bool(0x02af & b)));
Trade:: MeshData Magnum:: Primitives:: cubeWireframe()
#include <Magnum/Primitives/Cube.h>
Wireframe 3D cube.
2x2x2 cube, centered at origin. MeshPrimitive::
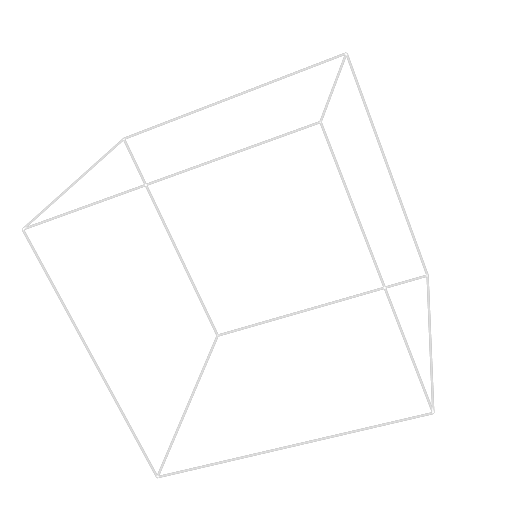
Trade:: MeshData Magnum:: Primitives:: cylinderSolid(UnsignedInt rings,
UnsignedInt segments,
Float halfLength,
CylinderFlags flags = {})
#include <Magnum/Primitives/Cylinder.h>
Solid 3D cylinder.
Parameters | |
---|---|
rings | Number of (face) rings. Must be larger or equal to 1 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
halfLength | Half the cylinder length |
flags | Flags |
Cylinder of radius 1.0f
along the Y axis, centered at origin. MeshPrimitive::
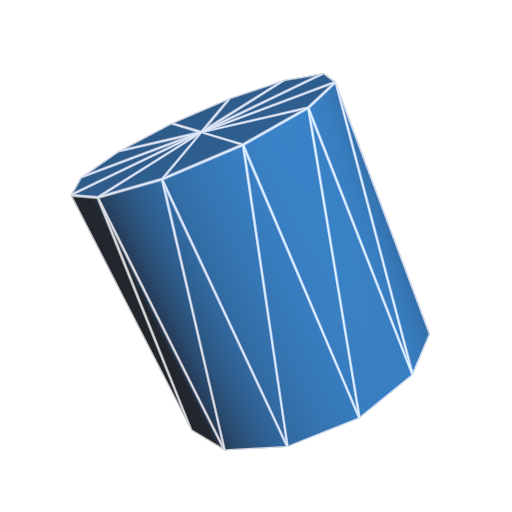
The cylinder is by default created with radius set to . In order to get radius , length and preserve correct normals, set halfLength
to and then scale all positions by , for example using MeshTools::
Trade:: MeshData Magnum:: Primitives:: cylinderWireframe(UnsignedInt rings,
UnsignedInt segments,
Float halfLength)
#include <Magnum/Primitives/Cylinder.h>
Wireframe 3D cylinder.
Parameters | |
---|---|
rings | Number of (line) rings. Must be larger or equal to 1 . |
segments | Number of (line) segments. Must be larger or equal to 4 and multiple of 4 . |
halfLength | Half the cylinder length |
Cylinder of radius 1.0f
along the Y axis, centerd at origin. MeshPrimitive::
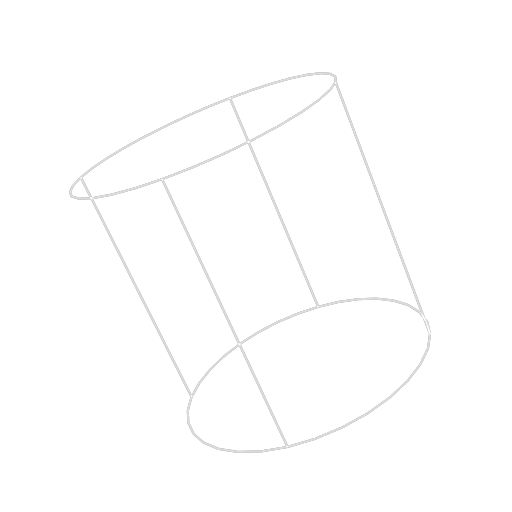
Trade:: MeshData Magnum:: Primitives:: gradient2D(const Vector2& a,
const Color4& colorA,
const Vector2& b,
const Color4& colorB)
#include <Magnum/Primitives/Gradient.h>
2D square with a gradient
2x2 square with vertex colors, centered at origin. Non-indexed MeshPrimitive::a
and b
, linearly interpolated from colorA
to colorB
.
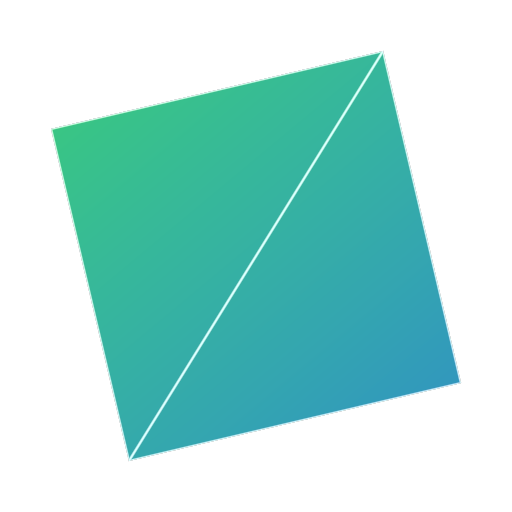
Trade:: MeshData Magnum:: Primitives:: gradient2DHorizontal(const Color4& colorLeft,
const Color4& colorRight)
#include <Magnum/Primitives/Gradient.h>
2D square with a horizontal gradient
Equivalent to calling gradient2D() like this:
Primitives::gradient2D({-1.0f, 0.0f}, colorLeft, {1.0f, 0.0f}, colorRight);
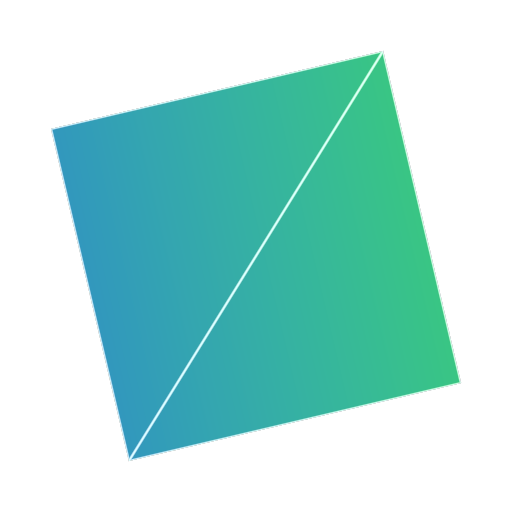
Trade:: MeshData Magnum:: Primitives:: gradient2DVertical(const Color4& colorBottom,
const Color4& colorTop)
#include <Magnum/Primitives/Gradient.h>
2D square with a vertical gradient
Equivalent to calling gradient2D() like this:
Primitives::gradient2D({0.0f, -1.0f}, colorBottom, {0.0f, 1.0f}, colorTop);
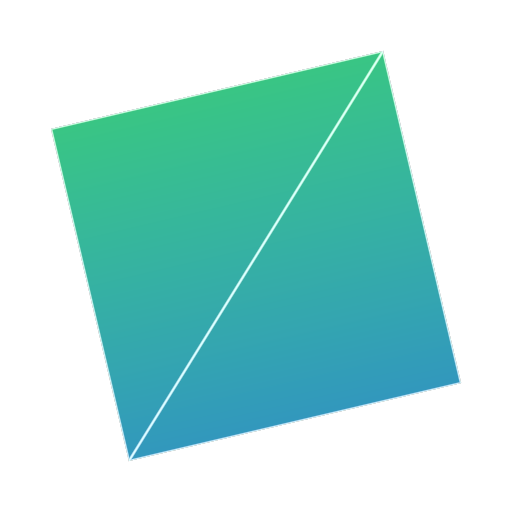
Trade:: MeshData Magnum:: Primitives:: gradient3D(const Vector3& a,
const Color4& colorA,
const Vector3& b,
const Color4& colorB)
#include <Magnum/Primitives/Gradient.h>
3D plane with a gradient
2x2 square on the XY plane with vertex colors, centered at origin. Non-indexed MeshPrimitive::a
and b
, linearly interpolated from colorA
to colorB
.
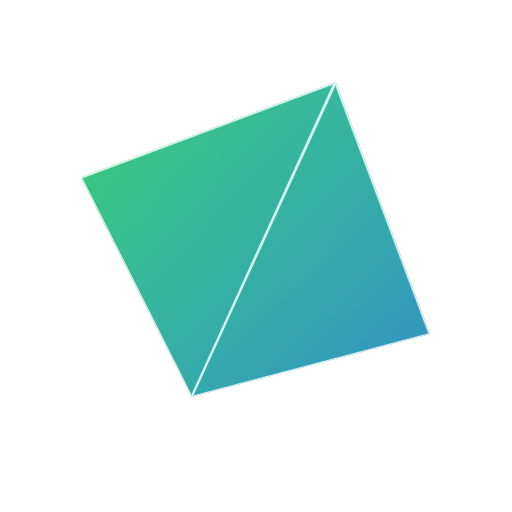
Trade:: MeshData Magnum:: Primitives:: gradient3DHorizontal(const Color4& colorLeft,
const Color4& colorRight)
#include <Magnum/Primitives/Gradient.h>
3D plane with a horizontal gradient
Equivalent to calling gradient3D() like this:
Primitives::gradient3D({-1.0f, 0.0f, 0.0f}, colorLeft, { 1.0f, 0.0f, 0.0f}, colorRight);
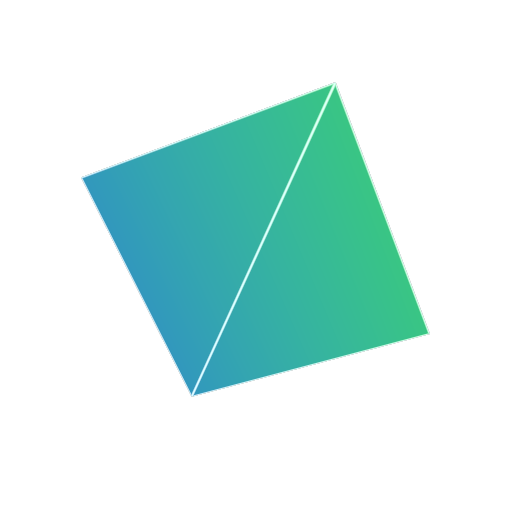
Trade:: MeshData Magnum:: Primitives:: gradient3DVertical(const Color4& colorBottom,
const Color4& colorTop)
#include <Magnum/Primitives/Gradient.h>
3D plane with a vertical gradient
Equivalent to calling gradient3D() like this:
Primitives::gradient3D({0.0f, -1.0f, 0.0f}, colorBottom, {0.0f, 1.0f, 0.0f}, colorTop);
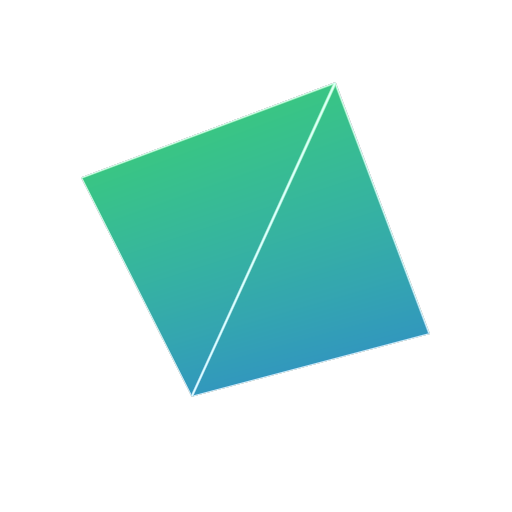
Trade:: MeshData Magnum:: Primitives:: grid3DSolid(const Vector2i& subdivisions,
GridFlags flags = GridFlag:: Normals)
#include <Magnum/Primitives/Grid.h>
Solid 3D grid.
2x2 grid on the XY plane with normals in positive Z direction, centered at origin. MeshPrimitive::
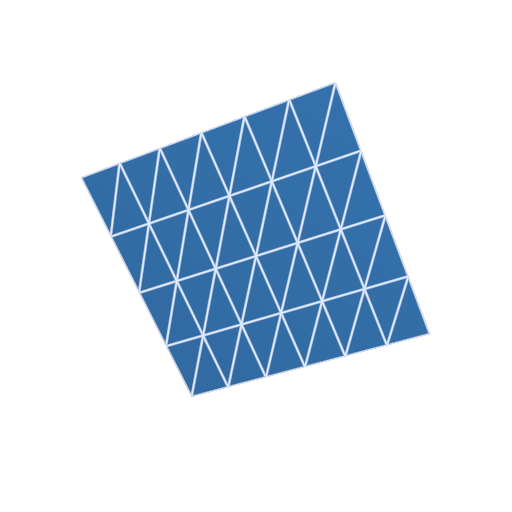
The subdivisions
parameter describes how many times the plane gets cut in each direction. Specifying {0, 0}
will make the result an (indexed) equivalent to planeSolid(); {5, 3}
will make the grid have 6 cells horizontally and 4 vertically. In particular, this is different from the subdivisions
parameter in icosphereSolid().
Trade:: MeshData Magnum:: Primitives:: grid3DWireframe(const Vector2i& subdivisions)
#include <Magnum/Primitives/Grid.h>
Wireframe 3D grid.
2x2 grid on the XY plane, centered at origin. MeshPrimitive::
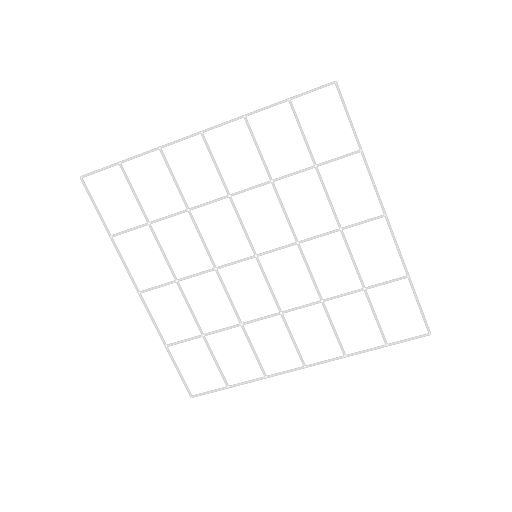
The subdivisions
parameter describes how many times the plane gets cut in each direction. Specifying {0, 0}
will make the result an (indexed) equivalent to planeWireframe(); {5, 3}
will make the grid have 6 cells horizontally and 4 vertically. In particular, this is different from the subdivisions
parameter in icosphereSolid(). Also please note the grid has vertices in each intersection to be suitable for deformation along the Z axis — not just long lines crossing each other.
Trade:: MeshData Magnum:: Primitives:: icosphereSolid(UnsignedInt subdivisions)
#include <Magnum/Primitives/Icosphere.h>
Solid 3D icosphere.
Parameters | |
---|---|
subdivisions | Number of subdivisions |
Sphere of radius 1.0f
, centered at origin. MeshPrimitive::
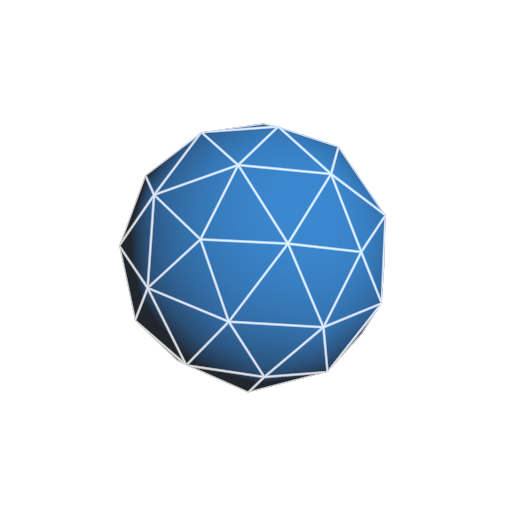
The subdivisions
parameter describes how many times is each icosphere triangle subdivided, recursively. Specifying 0
will result in an icosphere with 12 vertices and 20 faces, saying 1
will result in an icosphere with 80 faces (each triangle subdivided into four smaller), saying 2
will result in 320 faces and so on. In particular, this is different from the subdivisions
parameter in grid3DSolid() or grid3DWireframe().
Trade:: MeshData Magnum:: Primitives:: icosphereWireframe() new in 2020.06
#include <Magnum/Primitives/Icosphere.h>
Wireframe 3D icosphere.
Sphere of radius 1.0f
with 12 vertices and 30 edges, centered at origin. MeshPrimitive::
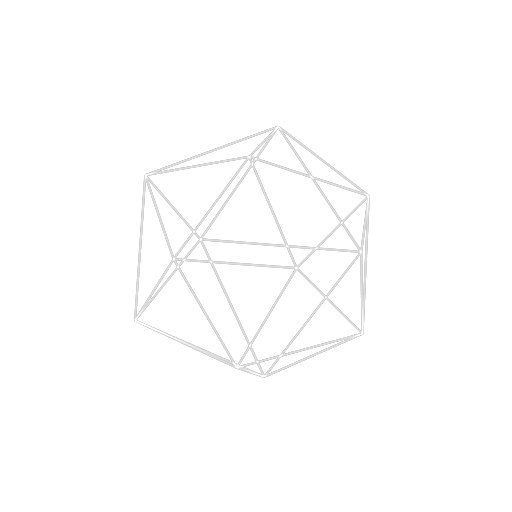
Trade:: MeshData Magnum:: Primitives:: line2D(const Vector2& a,
const Vector2& b)
#include <Magnum/Primitives/Line.h>
2D line
Non-indexed MeshPrimitive::a
to b
.
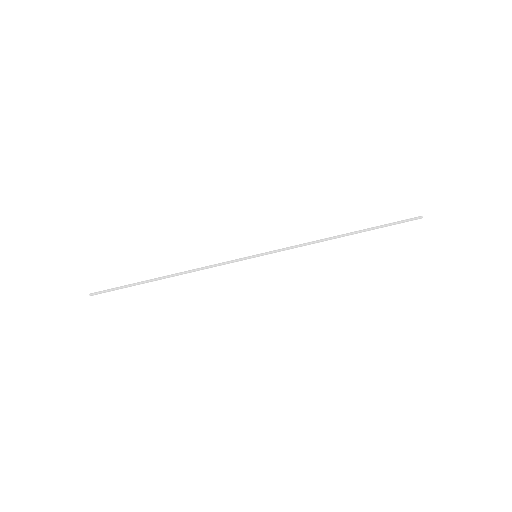
Trade:: MeshData Magnum:: Primitives:: line2D()
#include <Magnum/Primitives/Line.h>
2D line in an identity transformation
Equivalent to calling line2D(const Vector2&, const Vector2&) as
Primitives::line2D({0.0f, 0.0f}, {1.0f, 0.0f});
Trade:: MeshData Magnum:: Primitives:: line3D(const Vector3& a,
const Vector3& b)
#include <Magnum/Primitives/Line.h>
3D line
Non-indexed MeshPrimitive::a
to b
.
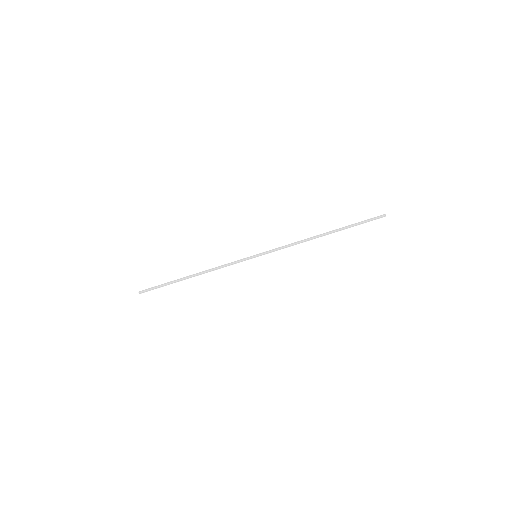
Trade:: MeshData Magnum:: Primitives:: line3D()
#include <Magnum/Primitives/Line.h>
3D line in an identity transformation
Unit-size line in direction of positive X axis. Equivalent to calling line3D(const Vector3&, const Vector3&) as
Primitives::line3D({0.0f, 0.0f, 0.0f}, {1.0f, 0.0f, 0.0f});
Trade:: MeshData Magnum:: Primitives:: planeSolid(PlaneFlags flags) new in 2020.06
#include <Magnum/Primitives/Plane.h>
Solid 3D plane.
Parameters | |
---|---|
flags | Flags |
2x2 square on the XY plane, centered at origin. Non-indexed MeshPrimitive::
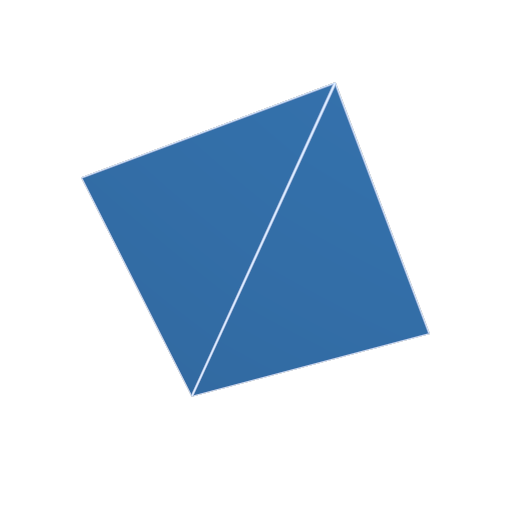
Trade:: MeshData Magnum:: Primitives:: planeSolid()
#include <Magnum/Primitives/Plane.h>
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Trade:: MeshData Magnum:: Primitives:: planeSolid(PlaneTextureCoords textureCoords)
#include <Magnum/Primitives/Plane.h>
Solid 3D plane.
Trade:: MeshData Magnum:: Primitives:: planeWireframe()
#include <Magnum/Primitives/Plane.h>
Wireframe 3D plane.
2x2 square on the XY plane, centered at origin. Non-indexed MeshPrimitive::
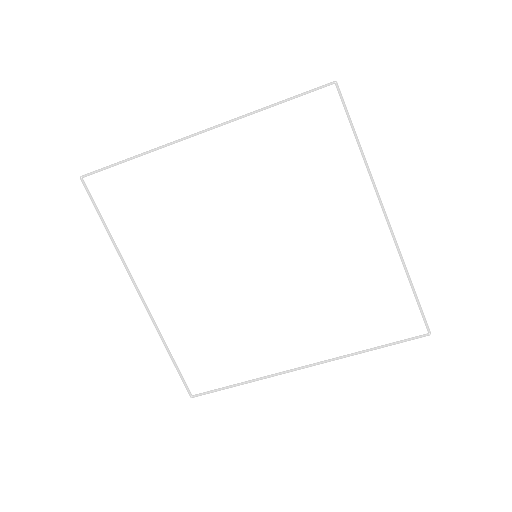
Trade:: MeshData Magnum:: Primitives:: squareSolid(SquareFlags flags = {}) new in 2020.06
#include <Magnum/Primitives/Square.h>
Solid 2D square.
Parameters | |
---|---|
flags | Flags |
2x2 square, centered at origin. Non-indexed MeshPrimitive::
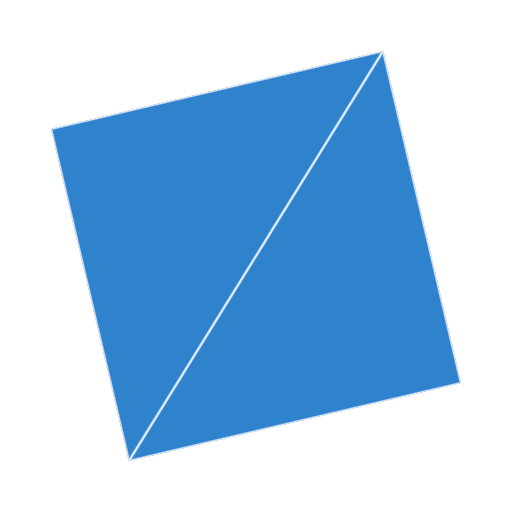
Trade:: MeshData Magnum:: Primitives:: squareSolid(SquareTextureCoords textureCoords)
#include <Magnum/Primitives/Square.h>
Solid 3D plane.
Trade:: MeshData Magnum:: Primitives:: squareWireframe()
#include <Magnum/Primitives/Square.h>
Wireframe 2D square.
2x2 square, centered at origin. Non-indexed MeshPrimitive::
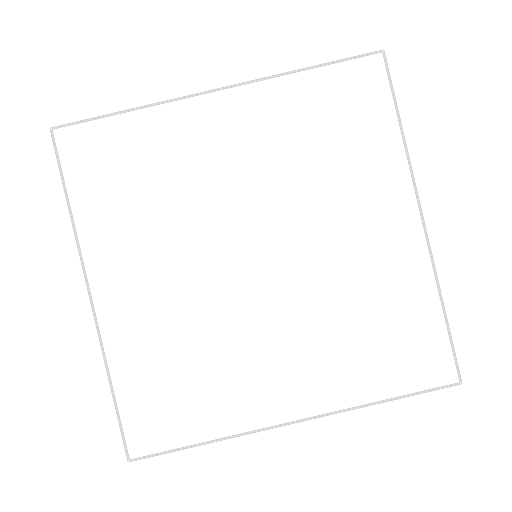
Trade:: MeshData Magnum:: Primitives:: uvSphereSolid(UnsignedInt rings,
UnsignedInt segments,
UVSphereFlags flags = {}) new in 2020.06
#include <Magnum/Primitives/UVSphere.h>
Solid 3D UV sphere.
Parameters | |
---|---|
rings | Number of (face) rings. Must be larger or equal to 2 . |
segments | Number of (face) segments. Must be larger or equal to 3 . |
flags | Flags |
Sphere of radius 1.0f
, centered at origin. MeshPrimitive::
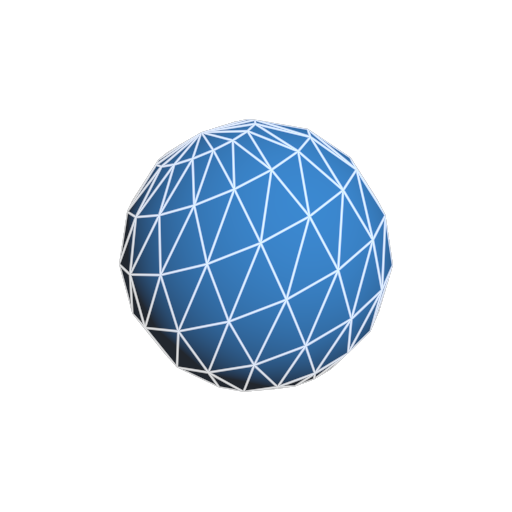
Trade:: MeshData Magnum:: Primitives:: uvSphereSolid(UnsignedInt rings,
UnsignedInt segments,
UVSphereTextureCoords textureCoords)
#include <Magnum/Primitives/UVSphere.h>
Solid 3D UV sphere.
Trade:: MeshData Magnum:: Primitives:: uvSphereWireframe(UnsignedInt rings,
UnsignedInt segments)
#include <Magnum/Primitives/UVSphere.h>
Wireframe 3D UV sphere.
Parameters | |
---|---|
rings | Number of (line) rings. Must be larger or equal to 2 and multiple of 2 . |
segments | Number of (line) segments. Must be larger or equal to 4 and multiple of 4 . |
Sphere of radius 1.0f
, centered at origin. MeshPrimitive::
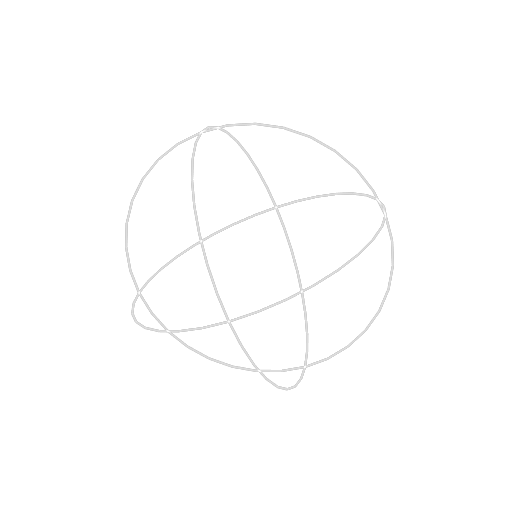