#include <Magnum/Shaders/VectorGL.h>
template<UnsignedInt dimensions>
VectorGL class new in Git master
Vector OpenGL shader.
Renders vector art in plain grayscale form. See also DistanceFieldVectorGL for more advanced effects. For rendering an unchanged texture you can use the FlatGL shader. You need to provide the Position and TextureCoordinates attributes in your triangle mesh and call at least bindVectorTexture(). By default, the shader renders the texture with a white color in an identity transformation. Use setTransformationProjectionMatrix(), setColor() and others to configure the shader.
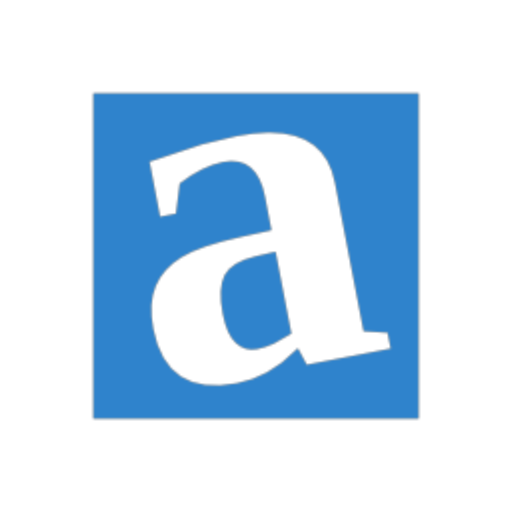
Alpha / transparency is supported by the shader implicitly, but to have it working on the framebuffer, you need to enable GL::
Example usage
Common mesh setup:
struct Vertex { Vector2 position; Vector2 textureCoordinates; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::VectorGL2D::Position{}, Shaders::VectorGL2D::TextureCoordinates{});
Common rendering setup:
Matrix3 transformationMatrix, projectionMatrix; GL::Texture2D texture; Shaders::VectorGL2D shader; shader.setColor(0x2f83cc_rgbf) .bindVectorTexture(texture) .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) .draw(mesh);
Uniform buffers
See Using uniform buffers for a high-level overview that applies to all shaders. In this particular case, because the shader doesn't need a separate projection and transformation matrix, a combined one is supplied via a TransformationProjectionUniform2D / TransformationProjectionUniform3D buffer bound with bindTransformationProjectionBuffer(). To maximize use of the limited uniform buffer memory, materials are supplied separately in a VectorMaterialUniform buffer bound with bindMaterialBuffer() and then referenced via materialId from a VectorDrawUniform buffer bound with bindDrawBuffer(); for optional texture transformation a per-draw TextureTransformationUniform buffer bound with bindTextureTransformationBuffer() can be supplied as well. A uniform buffer setup equivalent to the above would look like this:
GL::Buffer transformationProjectionUniform, materialUniform, drawUniform; transformationProjectionUniform.setData({ Shaders::TransformationProjectionUniform2D{} .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) }); materialUniform.setData({ Shaders::VectorMaterialUniform{} .setColor(0x2f83cc_rgbf) }); drawUniform.setData({ Shaders::VectorDrawUniform{} .setMaterialId(0) }); Shaders::VectorGL2D shader{Shaders::VectorGL2D::Configuration{} .setFlags(Shaders::VectorGL2D::Flag::UniformBuffers)}; shader .bindTransformationProjectionBuffer(transformationProjectionUniform) .bindMaterialBuffer(materialUniform) .bindDrawBuffer(drawUniform) .bindVectorTexture(texture) .draw(mesh);
For a multidraw workflow enable Flag::
Base classes
- class Magnum::GL::AbstractShaderProgram
- Base for shader program implementations.
Derived classes
- class Magnum::Shaders::VectorGL::CompileState new in Git master
- Asynchronous compilation state.
Public types
- class CompileState new in Git master
- Asynchronous compilation state.
- class Configuration new in Git master
- Configuration.
- enum (anonymous): UnsignedInt { ColorOutput = GenericGL<dimensions>::ColorOutput }
- enum class Flag: UnsignedByte { TextureTransformation = 1 << 0 new in 2020.06, UniformBuffers = 1 << 1 new in Git master, ShaderStorageBuffers = UniformBuffers|(1 << 3) new in Git master, MultiDraw = UniformBuffers|(1 << 2) new in Git master } new in 2020.06
- Flag.
- using Position = GenericGL<dimensions>::Position
- Vertex position.
- using TextureCoordinates = GenericGL<dimensions>::TextureCoordinates
- 2D texture coordinates
-
using Flags = Containers::
EnumSet<Flag> new in 2020.06 - Flags.
Public static functions
- static auto compile(const Configuration& configuration = Configuration{}) -> CompileState new in Git master
- Compile asynchronously.
- static auto compile(Flags flags) -> CompileState deprecated in Git master
- Compile asynchronously.
- static auto compile(Flags flags, UnsignedInt materialCount, UnsignedInt drawCount) -> CompileState deprecated in Git master
- Compile for a multi-draw scenario asynchronously.
Constructors, destructors, conversion operators
- VectorGL(const Configuration& configuration = Configuration{}) explicit new in Git master
- Constructor.
- VectorGL(Flags flags) deprecated in Git master explicit
- Constructor.
- VectorGL(Flags flags, UnsignedInt materialCount, UnsignedInt drawCount) deprecated in Git master explicit
- Construct for a multi-draw scenario.
- VectorGL(CompileState&& state) explicit new in Git master
- Finalize an asynchronous compilation.
- VectorGL(NoCreateT) explicit noexcept
- Construct without creating the underlying OpenGL object.
- VectorGL(const VectorGL<dimensions>&) deleted
- Copying is not allowed.
- VectorGL(VectorGL<dimensions>&&) defaulted noexcept
- Move constructor.
Public functions
- auto operator=(const VectorGL<dimensions>&) -> VectorGL<dimensions>& deleted
- Copying is not allowed.
- auto operator=(VectorGL<dimensions>&&) -> VectorGL<dimensions>& defaulted noexcept
- Move assignment.
- auto flags() const -> Flags new in 2020.06
- Flags.
- auto materialCount() const -> UnsignedInt new in Git master
- Material count.
- auto drawCount() const -> UnsignedInt new in Git master
- Draw count.
Uniform setters
Used only if Flag::
- auto setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix) -> VectorGL<dimensions>&
- Set transformation and projection matrix.
- auto setTextureMatrix(const Matrix3& matrix) -> VectorGL<dimensions>& new in 2020.06
- Set texture coordinate transformation matrix.
- auto setBackgroundColor(const Color4& color) -> VectorGL<dimensions>&
- Set background color.
- auto setColor(const Color4& color) -> VectorGL<dimensions>&
- Set fill color.
Texture binding
-
auto bindVectorTexture(GL::
Texture2D& texture) -> VectorGL<dimensions>& - Bind a vector texture.
Enum documentation
template<UnsignedInt dimensions>
enum Magnum:: Shaders:: VectorGL<dimensions>:: (anonymous): UnsignedInt
Enumerators | |
---|---|
ColorOutput |
Color shader output. Generic output, present always. Expects three- or four-component floating-point or normalized buffer attachment. |
template<UnsignedInt dimensions>
enum class Magnum:: Shaders:: VectorGL<dimensions>:: Flag: UnsignedByte new in 2020.06
Flag.
Enumerators | |
---|---|
TextureTransformation new in 2020.06 |
Enable texture coordinate transformation. |
UniformBuffers new in Git master |
Use uniform buffers. Expects that uniform data are supplied via bindTransformationProjectionBuffer(), bindDrawBuffer(), bindTextureTransformationBuffer() and bindMaterialBuffer() instead of direct uniform setters. |
ShaderStorageBuffers new in Git master |
Use shader storage buffers. Superset of functionality provided by Flag:: |
MultiDraw new in Git master |
Enable multidraw functionality. Implies Flag:: |
Typedef documentation
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::Position Magnum:: Shaders:: VectorGL<dimensions>:: Position
Vertex position.
Generic attribute, Vector2 in 2D, Vector3 in 3D.
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::TextureCoordinates Magnum:: Shaders:: VectorGL<dimensions>:: TextureCoordinates
2D texture coordinates
template<UnsignedInt dimensions>
typedef Containers:: EnumSet<Flag> Magnum:: Shaders:: VectorGL<dimensions>:: Flags new in 2020.06
Flags.
Function documentation
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: VectorGL<dimensions>:: compile(const Configuration& configuration = Configuration{}) new in Git master
Compile asynchronously.
Compared to VectorGL(const Configuration&) can perform an asynchronous compilation and linking. See Async shader compilation and linking for more information.
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: VectorGL<dimensions>:: compile(Flags flags)
Compile asynchronously.
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: VectorGL<dimensions>:: compile(Flags flags,
UnsignedInt materialCount,
UnsignedInt drawCount)
Compile for a multi-draw scenario asynchronously.
template<UnsignedInt dimensions>
Magnum:: Shaders:: VectorGL<dimensions>:: VectorGL(Flags flags) explicit
Constructor.
template<UnsignedInt dimensions>
Magnum:: Shaders:: VectorGL<dimensions>:: VectorGL(Flags flags,
UnsignedInt materialCount,
UnsignedInt drawCount) explicit
Construct for a multi-draw scenario.
template<UnsignedInt dimensions>
Magnum:: Shaders:: VectorGL<dimensions>:: VectorGL(CompileState&& state) explicit new in Git master
Finalize an asynchronous compilation.
Takes an asynchronous compilation state returned by compile() and forms a ready-to-use shader object. See Async shader compilation and linking for more information.
template<UnsignedInt dimensions>
Magnum:: Shaders:: VectorGL<dimensions>:: VectorGL(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to a moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active. However note that this is a low-level and a potentially dangerous API, see the documentation of NoCreate for alternatives.
template<UnsignedInt dimensions>
Flags Magnum:: Shaders:: VectorGL<dimensions>:: flags() const new in 2020.06
Flags.
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: VectorGL<dimensions>:: materialCount() const new in Git master
Material count.
Statically defined size of the VectorMaterialUniform uniform buffer bound with bindMaterialBuffer(). Has use only if Flag::
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: VectorGL<dimensions>:: drawCount() const new in Git master
Draw count.
Statically defined size of each of the TransformationProjectionUniform2D / TransformationProjectionUniform3D, VectorDrawUniform and TextureTransformationUniform uniform buffers bound with bindTransformationProjectionBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer(). Has use only if Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix)
Set transformation and projection matrix.
Returns | Reference to self (for method chaining) |
---|
Initial value is an identity matrix.
Expects that Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: setTextureMatrix(const Matrix3& matrix) new in 2020.06
Set texture coordinate transformation matrix.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
Expects that Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: setBackgroundColor(const Color4& color)
Set background color.
Returns | Reference to self (for method chaining) |
---|
Initial value is 0x00000000_rgbaf
.
Expects that Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: setColor(const Color4& color)
Set fill color.
Returns | Reference to self (for method chaining) |
---|
Initial value is 0xffffffff_rgbaf
.
Expects that Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: setDrawOffset(UnsignedInt offset) new in Git master
Set a draw offset.
Returns | Reference to self (for method chaining) |
---|
Specifies which item in the TransformationProjectionUniform2D / TransformationProjectionUniform3D, VectorDrawUniform and TextureTransformationUniform buffers bound with bindTransformationProjectionBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer() should be used for current draw. Expects that Flag::offset
is less than drawCount(). Initial value is 0
, if drawCount() is 1
, the function is a no-op as the shader assumes draw offset to be always zero.
If Flag::gl_DrawID
is added to this value, which makes each draw submitted via GL::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindTransformationProjectionBuffer(GL:: Buffer& buffer) new in Git master
Bind a transformation and projection uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindTransformationProjectionBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindDrawBuffer(GL:: Buffer& buffer) new in Git master
Bind a draw uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindDrawBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindTextureTransformationBuffer(GL:: Buffer& buffer) new in Git master
Bind a texture transformation uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that both Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindTextureTransformationBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindMaterialBuffer(GL:: Buffer& buffer) new in Git master
Bind a material uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindMaterialBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
VectorGL<dimensions>& Magnum:: Shaders:: VectorGL<dimensions>:: bindVectorTexture(GL:: Texture2D& texture)
Bind a vector texture.
Returns | Reference to self (for method chaining) |
---|
template<UnsignedInt dimensions>
template<UnsignedInt dimensions>
Debug& operator<<(Debug& debug,
VectorGL<dimensions>::Flag value)
Debug output operator.
template<UnsignedInt dimensions>
template<UnsignedInt dimensions>
Debug& operator<<(Debug& debug,
VectorGL<dimensions>::Flags value)
Debug output operator.