class new in Git master
#include <Magnum/Shaders/PhongGL.h>
PhongGL Phong OpenGL shader.
Uses ambient, diffuse and specular color or texture. For a colored mesh you need to provide the Position and Normal attributes in your triangle mesh. By default, the shader renders the mesh with a white color in an identity transformation. Use setProjectionMatrix(). setTransformationMatrix(), setNormalMatrix(), setLightPosition() and others to configure the shader.
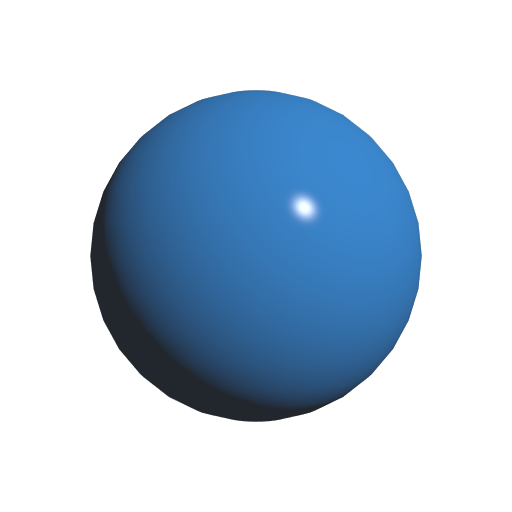
Colored rendering
Common mesh setup:
struct Vertex { Vector3 position; Vector3 normal; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::PhongGL::Position{}, Shaders::PhongGL::Normal{});
Common rendering setup:
Matrix4 transformationMatrix = Matrix4::translation(Vector3::zAxis(-5.0f)); Matrix4 projectionMatrix = Matrix4::perspectiveProjection(35.0_degf, 1.0f, 0.001f, 100.0f); Shaders::PhongGL shader; shader.setDiffuseColor(0x2f83cc_rgbf) .setShininess(200.0f) .setTransformationMatrix(transformationMatrix) .setNormalMatrix(transformationMatrix.normalMatrix()) .setProjectionMatrix(projectionMatrix) .draw(mesh);
Textured rendering
If you want to use textures, you need to provide also the TextureCoordinates attribute. Pass appropriate Flag combination to Configuration::
struct Vertex { Vector3 position; Vector3 normal; Vector2 textureCoordinates; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::PhongGL::Position{}, Shaders::PhongGL::Normal{}, Shaders::PhongGL::TextureCoordinates{});
Common rendering setup:
Matrix4 transformationMatrix, projectionMatrix; GL::Texture2D diffuseTexture, specularTexture; Shaders::PhongGL shader{Shaders::PhongGL::Configuration{} .setFlags(Shaders::PhongGL::Flag::DiffuseTexture| Shaders::PhongGL::Flag::SpecularTexture)}; shader.bindTextures(nullptr, &diffuseTexture, &specularTexture, nullptr) .setTransformationMatrix(transformationMatrix) .setNormalMatrix(transformationMatrix.normalMatrix()) .setProjectionMatrix(projectionMatrix) .draw(mesh);
Light specification
By default, the shader provides a single directional "fill" light, coming from the center of the camera. With Configuration::
Point lights are specified with camera-relative position and the last component set to
1.0f
together with setLightRanges() describing the attenuation. The range corresponds to the Trade::LightData:: range() and the attenuation is calculated as the following — see Attenuation calculation for more information: If you use Constants::
inf() as a range (which is also the default), the equation reduces down to a simple inverse square: Directional lights are specified with a camera-relative direction to the light with the last component set to
0.0f
— which effectively makes — and are not affected by values from setLightRanges() in any way:
Light color and intensity, corresponding to Trade::
The following example shows a three-light setup with one dim directional light shining from the top and two stronger but range-limited point lights:
Matrix4 directionalLight, pointLight1, pointLight2; // camera-relative Shaders::PhongGL shader{Shaders::PhongGL::Configuration{} .setLightCount(3)}; shader .setLightPositions({Vector4{directionalLight.up(), 0.0f}, Vector4{pointLight1.translation(), 1.0f}, Vector4{pointLight2.translation(), 1.0f}}) .setLightColors({0xf0f0ff_srgbf*0.1f, 0xff8080_srgbf*10.0f, 0x80ff80_srgbf*10.0f}) .setLightColors(…) .setLightRanges({Constants::inf(), 2.0f, 2.0f});
Ambient lights
In order to avoid redundant uniform inputs, there's no dedicated way to specify ambient lights. Instead, they are handled by the ambient color input, as the math for ambient color and lights is equivalent. Add the ambient colors together and reuse the diffuse texture in the bindAmbientTexture() slot to have it affected by the ambient as well:
Trade::LightData ambientLight = …; Shaders::PhongGL shader{Shaders::PhongGL::Configuration{} .setFlags(Shaders::PhongGL::Flag::AmbientTexture|…)}; shader .setAmbientColor(ambientColor + ambientLight.color()*ambientLight.intensity()) .bindAmbientTexture(diffuseTexture) .bindDiffuseTexture(diffuseTexture);
Zero lights
As a special case, creating this shader with zero lights makes its output equivalent to the FlatGL3D shader — only setAmbientColor() and bindAmbientTexture() (if Flag::
Alpha blending and masking
Alpha / transparency is supported by the shader implicitly, but to have it working on the framebuffer, you need to enable GL::
To avoid specular highlights on transparent areas, specular alpha should be always set to 0.0f
. On the other hand, non-zero specular alpha can be for example used to render transparent materials which are still expected to have specular highlights such as glass or soap bubbles.
An alternative is to enable Flag::discard
operation which is known to have considerable performance impact on some platforms. With proper depth sorting and blending you'll usually get much better performance and output quality.
For general alpha-masked drawing you need to provide an ambient texture with alpha channel and set alpha channel of the diffuse/specular color to 0.0f
so only ambient alpha will be taken into account. If you have a diffuse texture combined with the alpha mask, you can use that texture for both ambient and diffuse part and then separate the alpha like this:
Shaders::PhongGL shader{Shaders::PhongGL::Configuration{} .setFlags(Shaders::PhongGL::Flag::AmbientTexture| Shaders::PhongGL::Flag::DiffuseTexture)}; shader.bindTextures(&diffuseAlphaTexture, &diffuseAlphaTexture, nullptr, nullptr) .setAmbientColor(0x000000ff_rgbaf) .setDiffuseColor(Color4{diffuseRgb, 0.0f}) .setSpecularColor(Color4{specularRgb, 0.0f});
Normal mapping
If you want to use normal textures, enable Flag::
- either using a four-component Tangent4 attribute, where the sign of the fourth component defines handedness of tangent basis, as described in Trade::
MeshAttribute:: Tangent; - or a using pair of three-component Tangent and Bitangent attributes together with enabling Flag::
Bitangent
If you supply just a three-component Tangent attribute and no bitangents, the shader will implicitly assume the fourth component to be 1.0f
, forming a right-handed tangent space. This is a valid optimization when you have full control over the bitangent orientation, but won't work with general meshes.
The strength of the effect can be controlled by setNormalTextureScale(). See Trade::
Object ID output
The shader supports writing object ID to the framebuffer for object picking or other annotation purposes. Enable it using Flag::
The functionality is practically the same as in the FlatGL shader, see its documentation for more information and usage example.
Skinning
To render skinned meshes, bind up to two sets of up to four-component joint ID and weight attributes to JointIds / SecondaryJointIds and Weights / SecondaryWeights, set an appropriate joint count and per-vertex primary and secondary joint count in Configuration::
To avoid having to compile multiple shader variants for different joint matrix counts, set the maximum used joint count in Configuration::
Instanced rendering
Enabling Flag::
struct { Matrix4 transformation; Matrix3x3 normal; } instanceData[] { {Matrix4::translation({1.0f, 2.0f, 0.0f})*Matrix4::rotationX(90.0_degf), {}}, {Matrix4::translation({2.0f, 1.0f, 0.0f})*Matrix4::rotationY(90.0_degf), {}}, {Matrix4::translation({3.0f, 0.0f, 1.0f})*Matrix4::rotationZ(90.0_degf), {}}, // ... }; for(auto& instance: instanceData) instance.normal = instance.transformation.normalMatrix(); mesh.setInstanceCount(Containers::arraySize(instanceData)) .addVertexBufferInstanced(GL::Buffer{instanceData}, 1, 0, Shaders::PhongGL::TransformationMatrix{}, Shaders::PhongGL::NormalMatrix{});
For instanced skinning the joint buffer is assumed to contain joint transformations for all instances. By default all instances use the same joint transformations, seting setPerInstanceJointCount() will cause the shader to offset the per-vertex joint IDs with gl_InstanceID*perInstanceJointCount
.
Uniform buffers
See Using uniform buffers for a high-level overview that applies to all shaders. In this particular case, the shader needs a separate ProjectionUniform3D and TransformationUniform3D buffer bound with bindProjectionBuffer() and bindTransformationBuffer(), respectively, lights are supplied via a PhongLightUniform buffer bound with bindLightBuffer(). To maximize use of the limited uniform buffer memory, materials are supplied separately in a PhongMaterialUniform buffer bound with bindMaterialBuffer() and then referenced via materialId from a PhongDrawUniform bound with bindDrawBuffer(); for optional texture transformation a per-draw TextureTransformationUniform buffer bound with bindTextureTransformationBuffer() can be supplied as well. A uniform buffer setup equivalent to the colored case at the top, with one default light, would look like this:
GL::Buffer projectionUniform, lightUniform, materialUniform, transformationUniform, drawUniform; projectionUniform.setData({ Shaders::ProjectionUniform3D{} .setProjectionMatrix(projectionMatrix) }); lightUniform.setData({ Shaders::PhongLightUniform{} }); materialUniform.setData({ Shaders::PhongMaterialUniform{} .setDiffuseColor(0x2f83cc_rgbf) .setShininess(200.0f) }); transformationUniform.setData({ Shaders::TransformationUniform3D{} .setTransformationMatrix(transformationMatrix) }); drawUniform.setData({ Shaders::PhongDrawUniform{} .setNormalMatrix(transformationMatrix.normalMatrix()) .setMaterialId(0) }); Shaders::PhongGL shader{Shaders::PhongGL::Configuration{} .setFlags(Shaders::PhongGL::Flag::UniformBuffers)}; shader .bindProjectionBuffer(projectionUniform) .bindLightBuffer(lightUniform) .bindMaterialBuffer(materialUniform) .bindTransformationBuffer(transformationUniform) .bindDrawBuffer(drawUniform) .draw(mesh);
For a multidraw workflow enable Flag::
For skinning, joint matrices are supplied via a TransformationUniform3D buffer bound with bindJointBuffer(). In an instanced scenario the per-instance joint count is supplied via PhongDrawUniform::gl_InstanceID*perInstanceJointCount + jointOffset
. The setPerVertexJointCount() stays as an immediate uniform in the UBO and multidraw scenario as well, as it is tied to a particular mesh layout and thus doesn't need to vary per draw.
Base classes
- class Magnum::GL::AbstractShaderProgram
- Base for shader program implementations.
Derived classes
- class Magnum::Shaders::PhongGL::CompileState new in Git master
- Asynchronous compilation state.
Public types
- class CompileState new in Git master
- Asynchronous compilation state.
- class Configuration new in Git master
- Configuration.
- enum (anonymous): UnsignedInt { ColorOutput = GenericGL3D::ColorOutput new in 2019.10, ObjectIdOutput = GenericGL3D::ObjectIdOutput new in 2019.10 }
- enum class Flag: UnsignedInt { AmbientTexture = 1 << 0, DiffuseTexture = 1 << 1, SpecularTexture = 1 << 2, NormalTexture = 1 << 4 new in 2019.10, AlphaMask = 1 << 3, VertexColor = 1 << 5 new in 2019.10, DoubleSided = 1 << 20 new in Git master, Bitangent = 1 << 11 new in Git master, TextureTransformation = 1 << 6 new in 2020.06, ObjectId = 1 << 7 new in 2019.10, InstancedObjectId = (1 << 8)|ObjectId new in 2020.06, ObjectIdTexture = (1 << 17)|ObjectId new in Git master, InstancedTransformation = 1 << 9 new in 2020.06, InstancedTextureOffset = (1 << 10)|TextureTransformation new in 2020.06, UniformBuffers = 1 << 12 new in Git master, ShaderStorageBuffers = UniformBuffers|(1 << 19) new in Git master, MultiDraw = UniformBuffers|(1 << 13) new in Git master, TextureArrays = 1 << 14 new in Git master, LightCulling = 1 << 15 new in Git master, NoSpecular = 1 << 16 new in Git master, DynamicPerVertexJointCount = 1 << 18 new in Git master }
- Flag.
-
using Position = GenericGL3D::
Position - Vertex position.
-
using Normal = GenericGL3D::
Normal - Normal direction.
-
using Tangent = GenericGL3D::
Tangent new in 2019.10 - Tangent direction.
-
using Tangent4 = GenericGL3D::
Tangent4 new in Git master - Tangent direction with a bitangent sign.
-
using Bitangent = GenericGL3D::
Bitangent new in Git master - Bitangent direction.
-
using TextureCoordinates = GenericGL3D::
TextureCoordinates - 2D texture coordinates
-
using Color3 = GenericGL3D::
Color3 new in 2019.10 - Three-component vertex color.
-
using Color4 = GenericGL3D::
Color4 new in 2019.10 - Four-component vertex color.
-
using JointIds = GenericGL3D::
JointIds new in Git master - Joint ids.
-
using Weights = GenericGL3D::
Weights new in Git master - Weights.
-
using SecondaryJointIds = GenericGL3D::
SecondaryJointIds new in Git master - Secondary joint ids.
-
using SecondaryWeights = GenericGL3D::
SecondaryWeights new in Git master - Secondary weights.
-
using ObjectId = GenericGL3D::
ObjectId new in 2020.06 - (Instanced) object ID
-
using TransformationMatrix = GenericGL3D::
TransformationMatrix new in 2020.06 - (Instanced) transformation matrix
-
using NormalMatrix = GenericGL3D::
NormalMatrix new in 2020.06 - (Instanced) normal matrix
-
using TextureOffset = GenericGL3D::
TextureOffset new in 2020.06 - (Instanced) texture offset
-
using TextureOffsetLayer = GenericGL3D::
TextureOffsetLayer new in Git master - (Instanced) texture offset and layer
-
using Flags = Containers::
EnumSet<Flag> - Flags.
Public static functions
- static auto compile(const Configuration& configuration = Configuration{}) -> CompileState new in Git master
- Compile asynchronously.
- static auto compile(Flags flags, UnsignedInt lightCount = 1) -> CompileState deprecated in Git master
- Compile asynchronously.
- static auto compile(Flags flags, UnsignedInt lightCount, UnsignedInt materialCount, UnsignedInt drawCount) -> CompileState deprecated in Git master
- Compile for a multi-draw scenario asynchronously.
Constructors, destructors, conversion operators
- PhongGL(const Configuration& configuration = Configuration{}) explicit new in Git master
- Constructor.
- PhongGL(Flags flags, UnsignedInt lightCount = 1) deprecated in Git master explicit
- Constructor.
- PhongGL(Flags flags, UnsignedInt lightCount, UnsignedInt materialCount, UnsignedInt drawCount) deprecated in Git master explicit
- Construct for a multi-draw scenario.
- PhongGL(CompileState&& state) explicit new in Git master
- Finalize an asynchronous compilation.
- PhongGL(NoCreateT) explicit noexcept
- Construct without creating the underlying OpenGL object.
- PhongGL(const PhongGL&) deleted
- Copying is not allowed.
- PhongGL(PhongGL&&) defaulted noexcept
- Move constructor.
Public functions
- auto operator=(const PhongGL&) -> PhongGL& deleted
- Copying is not allowed.
- auto operator=(PhongGL&&) -> PhongGL& defaulted noexcept
- Move assignment.
- auto flags() const -> Flags
- Flags.
- auto lightCount() const -> UnsignedInt
- Light count.
- auto perDrawLightCount() const -> UnsignedInt new in Git master
- Per-draw light count.
- auto jointCount() const -> UnsignedInt new in Git master
- Joint count.
- auto perVertexJointCount() const -> UnsignedInt new in Git master
- Per-vertex joint count.
- auto secondaryPerVertexJointCount() const -> UnsignedInt new in Git master
- Secondary per-vertex joint count.
- auto materialCount() const -> UnsignedInt new in Git master
- Material count.
- auto drawCount() const -> UnsignedInt new in Git master
- Draw count.
- auto setPerVertexJointCount(UnsignedInt count, UnsignedInt secondaryCount = 0) -> PhongGL& new in Git master
- Set dynamic per-vertex skinning joint count.
Uniform setters
Used only if Flag::
-
auto setAmbientColor(const Magnum::
Color4& color) -> PhongGL& - Set ambient color.
-
auto setDiffuseColor(const Magnum::
Color4& color) -> PhongGL& - Set diffuse color.
- auto setNormalTextureScale(Float scale) -> PhongGL& new in Git master
- Set normal texture scale.
-
auto setSpecularColor(const Magnum::
Color4& color) -> PhongGL& - Set specular color.
- auto setShininess(Float shininess) -> PhongGL&
- Set shininess.
- auto setAlphaMask(Float mask) -> PhongGL&
- Set alpha mask value.
- auto setObjectId(UnsignedInt id) -> PhongGL&
- Set object ID.
- auto setTransformationMatrix(const Matrix4& matrix) -> PhongGL&
- Set transformation matrix.
- auto setNormalMatrix(const Matrix3x3& matrix) -> PhongGL&
- Set normal matrix.
- auto setProjectionMatrix(const Matrix4& matrix) -> PhongGL&
- Set projection matrix.
- auto setTextureMatrix(const Matrix3& matrix) -> PhongGL& new in 2020.06
- Set texture coordinate transformation matrix.
- auto setTextureLayer(UnsignedInt layer) -> PhongGL& new in Git master
- Set texture array layer.
-
auto setLightPositions(Containers::
ArrayView<const Vector4> positions) -> PhongGL& new in Git master - Set light positions.
-
auto setLightPositions(std::
initializer_list<Vector4> positions) -> PhongGL& new in Git master -
auto setLightPositions(Containers::
ArrayView<const Vector3> positions) -> PhongGL& deprecated in Git master - Set light positions.
-
auto setLightPositions(std::
initializer_list<Vector3> positions) -> PhongGL& deprecated in Git master - auto setLightPosition(UnsignedInt id, const Vector4& position) -> PhongGL& new in Git master
- Set position for given light.
- auto setLightPosition(UnsignedInt id, const Vector3& position) -> PhongGL& deprecated in Git master
- Set position for given light.
- auto setLightPosition(const Vector3& position) -> PhongGL& deprecated in Git master
- Set light position.
-
auto setLightColors(Containers::
ArrayView<const Magnum:: Color3> colors) -> PhongGL& new in Git master - Set light colors.
-
auto setLightColors(std::
initializer_list<Magnum:: Color3> colors) -> PhongGL& new in Git master -
auto setLightColors(Containers::
ArrayView<const Magnum:: Color4> colors) -> PhongGL& deprecated in Git master - Set light colors.
-
auto setLightColors(std::
initializer_list<Magnum:: Color4> colors) -> PhongGL& deprecated in Git master -
auto setLightColor(UnsignedInt id,
const Magnum::
Color3& color) -> PhongGL& new in Git master - Set color for given light.
-
auto setLightColor(UnsignedInt id,
const Magnum::
Color4& color) -> PhongGL& deprecated in Git master - Set color for given light.
-
auto setLightColor(const Magnum::
Color4& color) -> PhongGL& deprecated in Git master - Set light color.
-
auto setLightSpecularColors(Containers::
ArrayView<const Magnum:: Color3> colors) -> PhongGL& new in Git master - Set light specular colors.
-
auto setLightSpecularColors(std::
initializer_list<Magnum:: Color3> colors) -> PhongGL& new in Git master -
auto setLightSpecularColor(UnsignedInt id,
const Magnum::
Color3& color) -> PhongGL& new in Git master - Set specular color for given light.
-
auto setLightRanges(Containers::
ArrayView<const Float> ranges) -> PhongGL& new in Git master - Set light attenuation ranges.
-
auto setLightRanges(std::
initializer_list<Float> ranges) -> PhongGL& new in Git master - auto setLightRange(UnsignedInt id, Float range) -> PhongGL& new in Git master
- Set attenuation range for given light.
-
auto setJointMatrices(Containers::
ArrayView<const Matrix4> matrices) -> PhongGL& new in Git master - Set joint matrices.
-
auto setJointMatrices(std::
initializer_list<Matrix4> matrices) -> PhongGL& new in Git master - auto setJointMatrix(UnsignedInt id, const Matrix4& matrix) -> PhongGL& new in Git master
- Set joint matrix for given joint.
- auto setPerInstanceJointCount(UnsignedInt count) -> PhongGL& new in Git master
- Set per-instance joint count.
Texture binding
-
auto bindAmbientTexture(GL::
Texture2D& texture) -> PhongGL& - Bind an ambient texture.
-
auto bindAmbientTexture(GL::
Texture2DArray& texture) -> PhongGL& new in Git master - Bind an ambient array texture.
-
auto bindDiffuseTexture(GL::
Texture2D& texture) -> PhongGL& - Bind a diffuse texture.
-
auto bindDiffuseTexture(GL::
Texture2DArray& texture) -> PhongGL& new in Git master - Bind a diffuse array texture.
-
auto bindSpecularTexture(GL::
Texture2D& texture) -> PhongGL& - Bind a specular texture.
-
auto bindSpecularTexture(GL::
Texture2DArray& texture) -> PhongGL& new in Git master - Bind a specular array texture.
-
auto bindNormalTexture(GL::
Texture2D& texture) -> PhongGL& new in 2019.10 - Bind a normal texture.
-
auto bindNormalTexture(GL::
Texture2DArray& texture) -> PhongGL& new in Git master - Bind a normal array texture.
-
auto bindObjectIdTexture(GL::
Texture2D& texture) -> PhongGL& new in Git master - Bind an object ID texture.
-
auto bindObjectIdTexture(GL::
Texture2DArray& texture) -> PhongGL& new in Git master - Bind an object ID array texture.
-
auto bindTextures(GL::
Texture2D* ambient, GL:: Texture2D* diffuse, GL:: Texture2D* specular, GL:: Texture2D* normal = nullptr) -> PhongGL& - Bind textures.
Enum documentation
enum Magnum:: Shaders:: PhongGL:: (anonymous): UnsignedInt
Enumerators | |
---|---|
ColorOutput new in 2019.10 |
Color shader output. Generic output, present always. Expects three- or four-component floating-point or normalized buffer attachment. |
ObjectIdOutput new in 2019.10 |
Object ID shader output. Generic output, present only if Flag:: |
enum class Magnum:: Shaders:: PhongGL:: Flag: UnsignedInt
Flag.
Enumerators | |
---|---|
AmbientTexture |
Multiply ambient color with a texture. |
DiffuseTexture |
Multiply diffuse color with a texture. |
SpecularTexture |
Multiply specular color with a texture. |
NormalTexture new in 2019.10 |
Modify normals according to a texture. Requires the Tangent attribute to be present. |
AlphaMask |
Enable alpha masking. If the combined fragment color has an alpha less than the value specified with setAlphaMask(), given fragment is discarded. This uses the |
VertexColor new in 2019.10 |
Multiply the diffuse and ambient color with a vertex color. Requires either the Color3 or Color4 attribute to be present. |
DoubleSided new in Git master |
Double-sided rendering. By default, lighting is applied only to front-facing triangles, with back-facing triangles receiving just the ambient color or being culled away. If enabled, the shader will evaluate the lighting also on back-facing triangles with the normal flipped. Has no effect if no lights are used. Rendering back-facing triangles requires GL:: |
Bitangent new in Git master |
Use the separate Bitangent attribute for retrieving vertex bitangents. If this flag is not present, the last component of Tangent4 is used to calculate bitangent direction. See Normal mapping for more information. |
TextureTransformation new in 2020.06 |
Enable texture coordinate transformation. If this flag is set, the shader expects that at least one of Flag:: |
ObjectId new in 2019.10 |
Enable object ID output. See Object ID output for more information. |
InstancedObjectId new in 2020.06 |
Instanced object ID. Retrieves a per-instance / per-vertex object ID from the ObjectId attribute, outputting a sum of the per-vertex ID and ID coming from setObjectId() or PhongDrawUniform:: |
ObjectIdTexture new in Git master |
Object ID texture. Retrieves object IDs from a texture bound with bindObjectIdTexture(), outputting a sum of the object ID texture, the ID coming from setObjectId() or PhongDrawUniform:: |
InstancedTransformation new in 2020.06 |
Instanced transformation. Retrieves a per-instance transformation and normal matrix from the TransformationMatrix / NormalMatrix attributes and uses them together with matrices coming from setTransformationMatrix() and setNormalMatrix() or TransformationUniform3D:: |
InstancedTextureOffset new in 2020.06 |
Instanced texture offset. Retrieves a per-instance offset vector from the TextureOffset attribute and uses it together with the matrix coming from setTextureMatrix() or TextureTransformationUniform:: If Flag:: |
UniformBuffers new in Git master |
Use uniform buffers. Expects that uniform data are supplied via bindProjectionBuffer(), bindTransformationBuffer(), bindDrawBuffer(), bindTextureTransformationBuffer(), bindMaterialBuffer() and bindLightBuffer() instead of direct uniform setters. |
ShaderStorageBuffers new in Git master |
Use shader storage buffers. Superset of functionality provided by Flag:: |
MultiDraw new in Git master |
Enable multidraw functionality. Implies Flag:: |
TextureArrays new in Git master |
Use 2D texture arrays. Expects that the texture is supplied via bindAmbientTexture(GL:: |
LightCulling new in Git master |
Enable light culling in uniform buffer workflows using the PhongDrawUniform:: |
NoSpecular new in Git master |
Disable specular contribution in light calculation. Can result in a significant performance improvement compared to calling setSpecularColor() with |
DynamicPerVertexJointCount new in Git master |
Dynamic per-vertex joint count for skinning. Uses only the first M / N primary / secondary components defined by setPerVertexJointCount() instead of all primary / secondary components defined by Configuration:: |
Typedef documentation
typedef GenericGL3D:: Position Magnum:: Shaders:: PhongGL:: Position
Vertex position.
typedef GenericGL3D:: Normal Magnum:: Shaders:: PhongGL:: Normal
Normal direction.
Generic attribute, Vector3. Used only if perDrawLightCount() isn't 0
.
typedef GenericGL3D:: Tangent Magnum:: Shaders:: PhongGL:: Tangent new in 2019.10
Tangent direction.
Generic attribute, Vector3. Use either this or the Tangent4 attribute. If only a three-component attribute is used and Flag::1.0f
. Used only if Flag::0
.
typedef GenericGL3D:: Tangent4 Magnum:: Shaders:: PhongGL:: Tangent4 new in Git master
Tangent direction with a bitangent sign.
Generic attribute, Vector4. Use either this or the Tangent attribute. If Flag::0
.
typedef GenericGL3D:: Bitangent Magnum:: Shaders:: PhongGL:: Bitangent new in Git master
Bitangent direction.
Generic attribute, Vector3. Use either this or the Tangent4 attribute. Used only if both Flag::0
.
typedef GenericGL3D:: TextureCoordinates Magnum:: Shaders:: PhongGL:: TextureCoordinates
2D texture coordinates
Generic attribute, Vector2, used only if at least one of Flag::
typedef GenericGL3D:: Color3 Magnum:: Shaders:: PhongGL:: Color3 new in 2019.10
Three-component vertex color.
Generic attribute, Magnum::
typedef GenericGL3D:: Color4 Magnum:: Shaders:: PhongGL:: Color4 new in 2019.10
Four-component vertex color.
Generic attribute, Magnum::
typedef GenericGL3D:: JointIds Magnum:: Shaders:: PhongGL:: JointIds new in Git master
Joint ids.
Generic attribute, Vector4ui. Used only if perVertexJointCount() isn't 0
.
typedef GenericGL3D:: Weights Magnum:: Shaders:: PhongGL:: Weights new in Git master
Weights.
Generic attribute, Vector4. Used only if perVertexJointCount() isn't 0
.
typedef GenericGL3D:: SecondaryJointIds Magnum:: Shaders:: PhongGL:: SecondaryJointIds new in Git master
Secondary joint ids.
Generic attribute, Vector4ui. Used only if secondaryPerVertexJointCount() isn't 0
.
typedef GenericGL3D:: SecondaryWeights Magnum:: Shaders:: PhongGL:: SecondaryWeights new in Git master
Secondary weights.
Generic attribute, Vector4. Used only if secondaryPerVertexJointCount() isn't 0
.
typedef GenericGL3D:: ObjectId Magnum:: Shaders:: PhongGL:: ObjectId new in 2020.06
(Instanced) object ID
Generic attribute, UnsignedInt. Used only if Flag::
typedef GenericGL3D:: TransformationMatrix Magnum:: Shaders:: PhongGL:: TransformationMatrix new in 2020.06
(Instanced) transformation matrix
Generic attribute, Matrix4. Used only if Flag::
typedef GenericGL3D:: NormalMatrix Magnum:: Shaders:: PhongGL:: NormalMatrix new in 2020.06
(Instanced) normal matrix
Generic attribute, Matrix3x3. Used only if Flag::
typedef GenericGL3D:: TextureOffset Magnum:: Shaders:: PhongGL:: TextureOffset new in 2020.06
(Instanced) texture offset
Generic attribute, Vector2. Used only if Flag::
typedef GenericGL3D:: TextureOffsetLayer Magnum:: Shaders:: PhongGL:: TextureOffsetLayer new in Git master
(Instanced) texture offset and layer
Generic attribute, Vector3, with the last component interpreted as an integer. Use either this or the TextureOffset attribute. First two components used only if Flag::
typedef Containers:: EnumSet<Flag> Magnum:: Shaders:: PhongGL:: Flags
Flags.
Function documentation
static CompileState Magnum:: Shaders:: PhongGL:: compile(const Configuration& configuration = Configuration{}) new in Git master
Compile asynchronously.
Compared to PhongGL(const Configuration&) can perform an asynchronous compilation and linking. See Async shader compilation and linking for more information.
static CompileState Magnum:: Shaders:: PhongGL:: compile(Flags flags,
UnsignedInt lightCount = 1)
Compile asynchronously.
static CompileState Magnum:: Shaders:: PhongGL:: compile(Flags flags,
UnsignedInt lightCount,
UnsignedInt materialCount,
UnsignedInt drawCount)
Compile for a multi-draw scenario asynchronously.
Magnum:: Shaders:: PhongGL:: PhongGL(Flags flags,
UnsignedInt lightCount = 1) explicit
Constructor.
Magnum:: Shaders:: PhongGL:: PhongGL(Flags flags,
UnsignedInt lightCount,
UnsignedInt materialCount,
UnsignedInt drawCount) explicit
Construct for a multi-draw scenario.
Magnum:: Shaders:: PhongGL:: PhongGL(CompileState&& state) explicit new in Git master
Finalize an asynchronous compilation.
Takes an asynchronous compilation state returned by compile() and forms a ready-to-use shader object. See Async shader compilation and linking for more information.
Magnum:: Shaders:: PhongGL:: PhongGL(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to a moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active. However note that this is a low-level and a potentially dangerous API, see the documentation of NoCreate for alternatives.
UnsignedInt Magnum:: Shaders:: PhongGL:: lightCount() const
Light count.
If Flag::
If Flag::
UnsignedInt Magnum:: Shaders:: PhongGL:: perDrawLightCount() const new in Git master
Per-draw light count.
Number of lights out of lightCount() applied per draw. If Flag::0
, no lighting calculations are performed and only the ambient contribution to the color is used.
UnsignedInt Magnum:: Shaders:: PhongGL:: jointCount() const new in Git master
Joint count.
If Flag::
UnsignedInt Magnum:: Shaders:: PhongGL:: perVertexJointCount() const new in Git master
Per-vertex joint count.
Returns the value set with Configuration::
UnsignedInt Magnum:: Shaders:: PhongGL:: secondaryPerVertexJointCount() const new in Git master
Secondary per-vertex joint count.
Returns the value set with Configuration::
UnsignedInt Magnum:: Shaders:: PhongGL:: materialCount() const new in Git master
Material count.
Statically defined size of the PhongMaterialUniform uniform buffer bound with bindMaterialBuffer(). Has use only if Flag::
UnsignedInt Magnum:: Shaders:: PhongGL:: drawCount() const new in Git master
Draw count.
Statically defined size of each of the TransformationUniform3D, PhongDrawUniform and TextureTransformationUniform uniform buffers bound with bindTransformationBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer(). Has use only if Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setPerVertexJointCount(UnsignedInt count,
UnsignedInt secondaryCount = 0) new in Git master
Set dynamic per-vertex skinning joint count.
Returns | Reference to self (for method chaining) |
---|
Allows reducing the count of iterated joints for a particular draw call, making it possible to use a single shader with meshes that contain different count of per-vertex joints. See Flag::
Expects that Flag::count
is not larger than perVertexJointCount() and secondaryCount
not larger than secondaryPerVertexJointCount().
PhongGL& Magnum:: Shaders:: PhongGL:: setAmbientColor(const Magnum:: Color4& color)
Set ambient color.
Returns | Reference to self (for method chaining) |
---|
If Flag::0xffffffff_rgbaf
and the color will be multiplied with ambient texture, otherwise default value is 0x00000000_rgbaf
. If Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setDiffuseColor(const Magnum:: Color4& color)
Set diffuse color.
Returns | Reference to self (for method chaining) |
---|
Initial value is 0xffffffff_rgbaf
. If Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setNormalTextureScale(Float scale) new in Git master
Set normal texture scale.
Returns | Reference to self (for method chaining) |
---|
Affects strength of the normal mapping. Initial value is 1.0f
, meaning the normal texture is not changed in any way; a value of 0.0f
disables the normal texture effect altogether.
Expects that the shader was created with Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setSpecularColor(const Magnum:: Color4& color)
Set specular color.
Returns | Reference to self (for method chaining) |
---|
Initial value is 0xffffff00_rgbaf
. Expects that the shader was not created with Flag::0x00000000_rgbaf
. If perDrawLightCount() is zero, this function is a no-op, as specular color doesn't contribute to the output in that case.
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setShininess(Float shininess)
Set shininess.
Returns | Reference to self (for method chaining) |
---|
The larger value, the harder surface (smaller specular highlight). Initial value is 80.0f
. If perDrawLightCount() is zero, this function is a no-op, as specular color doesn't contribute to the output in that case.
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setAlphaMask(Float mask)
Set alpha mask value.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0.5f
. See the flag documentation for further information.
This corresponds to glAlphaFunc() in classic OpenGL.
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setObjectId(UnsignedInt id)
Set object ID.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0
. If Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setTransformationMatrix(const Matrix4& matrix)
Set transformation matrix.
Returns | Reference to self (for method chaining) |
---|
You need to set also setNormalMatrix() with a corresponding value. Initial value is an identity matrix. If Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setNormalMatrix(const Matrix3x3& matrix)
Set normal matrix.
Returns | Reference to self (for method chaining) |
---|
The matrix doesn't need to be normalized, as renormalization is done per-fragment anyway. You need to set also setTransformationMatrix() with a corresponding value. Initial value is an identity matrix. If perDrawLightCount() is zero, this function is a no-op, as normals don't contribute to the output in that case. If Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setProjectionMatrix(const Matrix4& matrix)
Set projection matrix.
Returns | Reference to self (for method chaining) |
---|
Initial value is an identity matrix (i.e., an orthographic projection of the default cube).
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setTextureMatrix(const Matrix3& matrix) new in 2020.06
Set texture coordinate transformation matrix.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setTextureLayer(UnsignedInt layer) new in Git master
Set texture array layer.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0
. If Flag::
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightPositions(Containers:: ArrayView<const Vector4> positions) new in Git master
Set light positions.
Returns | Reference to self (for method chaining) |
---|
Depending on the fourth component, the value is treated as either a camera-relative position of a point light, if the fourth component is 1.0f
; or a direction to a directional light, if the fourth component is 0.0f
. Expects that the size of the positions
array is the same as lightCount(). Initial values are {0.0f, 0.0f, 1.0f, 0.0f}
— a directional "fill" light coming from the camera.
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightPositions(std:: initializer_list<Vector4> positions) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightPositions(Containers:: ArrayView<const Vector3> positions)
Set light positions.
0.0f
to preserve the original behavior as close as possible.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightPositions(std:: initializer_list<Vector3> positions)
0.0f
to preserve the original behavior as close as possible.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightPosition(UnsignedInt id,
const Vector4& position) new in Git master
Set position for given light.
Returns | Reference to self (for method chaining) |
---|
Unlike setLightPositions() updates just a single light position. If updating more than one light, prefer the batch function instead to reduce the count of GL API calls. Expects that id
is less than lightCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightPosition(UnsignedInt id,
const Vector3& position)
Set position for given light.
0.0f
to preserve the original behavior as close as possible.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightPosition(const Vector3& position)
Set light position.
0.0f
to preserve the original behavior as close as possible.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightColors(Containers:: ArrayView<const Magnum:: Color3> colors) new in Git master
Set light colors.
Returns | Reference to self (for method chaining) |
---|
Initial values are 0xffffff_rgbf
. Expects that the size of the colors
array is the same as lightCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightColors(std:: initializer_list<Magnum:: Color3> colors) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightColors(Containers:: ArrayView<const Magnum:: Color4> colors)
Set light colors.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightColors(std:: initializer_list<Magnum:: Color4> colors)
PhongGL& Magnum:: Shaders:: PhongGL:: setLightColor(UnsignedInt id,
const Magnum:: Color3& color) new in Git master
Set color for given light.
Returns | Reference to self (for method chaining) |
---|
Unlike setLightColors() updates just a single light color. If updating more than one light, prefer the batch function instead to reduce the count of GL API calls. Expects that id
is less than lightCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightColor(UnsignedInt id,
const Magnum:: Color4& color)
Set color for given light.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightColor(const Magnum:: Color4& color)
Set light color.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightSpecularColors(Containers:: ArrayView<const Magnum:: Color3> colors) new in Git master
Set light specular colors.
Returns | Reference to self (for method chaining) |
---|
Usually you'd set this value to the same as setLightColors(), but it allows for greater flexibility such as disabling specular highlights on certain lights. Initial values are 0xffffff_rgbf
. Expects that the size of the colors
array is the same as lightCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightSpecularColors(std:: initializer_list<Magnum:: Color3> colors) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightSpecularColor(UnsignedInt id,
const Magnum:: Color3& color) new in Git master
Set specular color for given light.
Returns | Reference to self (for method chaining) |
---|
Unlike setLightSpecularColors() updates just a single light color. If updating more than one light, prefer the batch function instead to reduce the count of GL API calls. Expects that id
is less than lightCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightRanges(Containers:: ArrayView<const Float> ranges) new in Git master
Set light attenuation ranges.
Returns | Reference to self (for method chaining) |
---|
Initial values are Constants::ranges
array is the same as lightCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setLightRanges(std:: initializer_list<Float> ranges) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: setLightRange(UnsignedInt id,
Float range) new in Git master
Set attenuation range for given light.
Returns | Reference to self (for method chaining) |
---|
Unlike setLightRanges() updates just a single light range. If updating more than one light, prefer the batch function instead to reduce the count of GL API calls. Expects that id
is less than lightCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setJointMatrices(Containers:: ArrayView<const Matrix4> matrices) new in Git master
Set joint matrices.
Returns | Reference to self (for method chaining) |
---|
Initial values are identity transformations. Expects that the size of the matrices
array is the same as jointCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setJointMatrices(std:: initializer_list<Matrix4> matrices) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: setJointMatrix(UnsignedInt id,
const Matrix4& matrix) new in Git master
Set joint matrix for given joint.
Returns | Reference to self (for method chaining) |
---|
Unlike setJointMatrices() updates just a single joint matrix. Expects that id
is less than jointCount().
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setPerInstanceJointCount(UnsignedInt count) new in Git master
Set per-instance joint count.
Returns | Reference to self (for method chaining) |
---|
Offset added to joint IDs in the JointIds and SecondaryJointIds in instanced draws. Should be less than jointCount(). Initial value is 0
, meaning every instance will use the same joint matrices, setting it to a non-zero value causes the joint IDs to be interpreted as gl_InstanceID*count + jointId
.
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: setDrawOffset(UnsignedInt offset) new in Git master
Set a draw offset.
Returns | Reference to self (for method chaining) |
---|
Specifies which item in the TransformationUniform3D, PhongDrawUniform and TextureTransformationUniform buffers bound with bindTransformationBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer() should be used for current draw. Expects that Flag::offset
is less than drawCount(). Initial value is 0
, if drawCount() is 1
, the function is a no-op as the shader assumes draw offset to be always zero.
If Flag::gl_DrawID
is added to this value, which makes each draw submitted via GL::
PhongGL& Magnum:: Shaders:: PhongGL:: bindProjectionBuffer(GL:: Buffer& buffer) new in Git master
Bind a projection uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindProjectionBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: bindTransformationBuffer(GL:: Buffer& buffer) new in Git master
Bind a transformation uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindTransformationBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: bindDrawBuffer(GL:: Buffer& buffer) new in Git master
Bind a draw uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindDrawBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: bindTextureTransformationBuffer(GL:: Buffer& buffer) new in Git master
Bind a texture transformation uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that both Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindTextureTransformationBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: bindMaterialBuffer(GL:: Buffer& buffer) new in Git master
Bind a material uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindMaterialBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: bindLightBuffer(GL:: Buffer& buffer) new in Git master
Bind a light uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindLightBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: bindJointBuffer(GL:: Buffer& buffer) new in Git master
Bind a joint matrix uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindJointBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
PhongGL& Magnum:: Shaders:: PhongGL:: bindAmbientTexture(GL:: Texture2D& texture)
Bind an ambient texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindAmbientTexture(GL:: Texture2DArray& texture) new in Git master
Bind an ambient array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with both Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindDiffuseTexture(GL:: Texture2D& texture)
Bind a diffuse texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindDiffuseTexture(GL:: Texture2DArray& texture) new in Git master
Bind a diffuse array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with both Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindSpecularTexture(GL:: Texture2D& texture)
Bind a specular texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindSpecularTexture(GL:: Texture2DArray& texture) new in Git master
Bind a specular array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with both Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindNormalTexture(GL:: Texture2D& texture) new in 2019.10
Bind a normal texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindNormalTexture(GL:: Texture2DArray& texture) new in Git master
Bind a normal array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with both Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindObjectIdTexture(GL:: Texture2D& texture) new in Git master
Bind an object ID texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindObjectIdTexture(GL:: Texture2DArray& texture) new in Git master
Bind an object ID array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with both Flag::
PhongGL& Magnum:: Shaders:: PhongGL:: bindTextures(GL:: Texture2D* ambient,
GL:: Texture2D* diffuse,
GL:: Texture2D* specular,
GL:: Texture2D* normal = nullptr)
Bind textures.
Returns | Reference to self (for method chaining) |
---|
A particular texture has effect only if particular texture flag from Flag is set, you can use nullptr
for the rest. Expects that the shader was created with at least one of Flag::
Debug& operator<<(Debug& debug,
PhongGL:: Flag value)
Debug output operator.
Debug& operator<<(Debug& debug,
PhongGL:: Flags value)
Debug output operator.