template<UnsignedInt dimensions>
DistanceFieldVectorGL class new in Git master
Distance field vector OpenGL shader.
Renders vector graphics in a form of signed distance field. See TextureTools::
You need to provide Position and TextureCoordinates attributes in your triangle mesh and call at least bindVectorTexture(). By default, the shader renders the distance field texture with a white color in an identity transformation, use setTransformationProjectionMatrix(), setColor() and others to configure the shader. Edge smoothness can be controlled using setSmoothness().
Alpha / transparency is supported by the shader implicitly, but to have it working on the framebuffer, you need to enable GL::
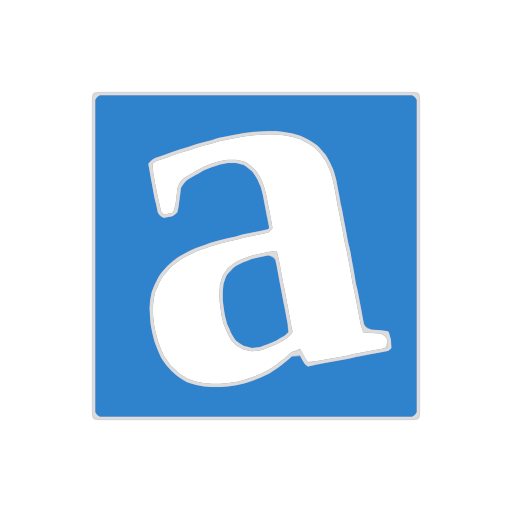
Example usage
Common mesh setup:
struct { Vector2 position; Vector2 textureCoordinates; } vertices[]{ … }; GL::Mesh mesh; mesh.addVertexBuffer(GL::Buffer{vertices}, 0, Shaders::DistanceFieldVectorGL2D::Position{}, Shaders::DistanceFieldVectorGL2D::TextureCoordinates{}) .setCount(Containers::arraySize(vertices));
Common rendering setup:
Matrix3 transformationMatrix, projectionMatrix; GL::Texture2D texture; Shaders::DistanceFieldVectorGL2D shader; shader.setColor(0x2f83cc_rgbf) .setOutlineColor(0xdcdcdc_rgbf) .setOutlineRange(0.6f, 0.4f) .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) .bindVectorTexture(texture) .draw(mesh);
If Flag::0
is picked. Additionally, the value of setTextureLayer(), which is 0
by default, is added to the layer.
Uniform buffers
See Using uniform buffers for a high-level overview that applies to all shaders. In this particular case, because the shader doesn't need a separate projection and transformation matrix, a combined one is supplied via a TransformationProjectionUniform2D / TransformationProjectionUniform3D buffer bound with bindTransformationProjectionBuffer(). To maximize use of the limited uniform buffer memory, materials are supplied separately in a DistanceFieldVectorMaterialUniform buffer bound with bindMaterialBuffer() and then referenced via materialId from a DistanceFieldVectorDrawUniform bound with bindDrawBuffer(); for optional texture transformation a per-draw TextureTransformationUniform buffer bound with bindTextureTransformationBuffer() can be supplied as well. A uniform buffer setup equivalent to the above would look like this:
GL::Buffer transformationProjectionUniform, materialUniform, drawUniform; transformationProjectionUniform.setData({ Shaders::TransformationProjectionUniform2D{} .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) }); materialUniform.setData({ Shaders::DistanceFieldVectorMaterialUniform{} .setColor(0x2f83cc_rgbf) .setOutlineColor(0xdcdcdc_rgbf) .setOutlineRange(0.6f, 0.4f) }); drawUniform.setData({ Shaders::DistanceFieldVectorDrawUniform{} .setMaterialId(0) }); Shaders::DistanceFieldVectorGL2D shader{ Shaders::DistanceFieldVectorGL2D::Configuration{} .setFlags(Shaders::DistanceFieldVectorGL2D::Flag::UniformBuffers)}; shader .bindTransformationProjectionBuffer(transformationProjectionUniform) .bindMaterialBuffer(materialUniform) .bindDrawBuffer(drawUniform) .bindVectorTexture(texture) .draw(mesh);
For a multidraw workflow enable Flag::
Base classes
- class Magnum::GL::AbstractShaderProgram
- Base for shader program implementations.
Derived classes
- class Magnum::Shaders::DistanceFieldVectorGL::CompileState new in Git master
- Asynchronous compilation state.
Public types
- class CompileState new in Git master
- Asynchronous compilation state.
- class Configuration new in Git master
- Configuration.
- enum (anonymous): UnsignedInt { ColorOutput = GenericGL<dimensions>::ColorOutput }
- enum class Flag: UnsignedByte { TextureTransformation = 1 << 0 new in 2020.06, UniformBuffers = 1 << 1 new in Git master, ShaderStorageBuffers = UniformBuffers|(1 << 3) new in Git master, MultiDraw = UniformBuffers|(1 << 2) new in Git master, TextureArrays = 1 << 4 new in Git master } new in 2020.06
- Flag.
- using Position = GenericGL<dimensions>::Position
- Vertex position.
- using TextureCoordinates = GenericGL<dimensions>::TextureCoordinates
- 2D texture coordinates
- using TextureArrayCoordinates = GenericGL<dimensions>::TextureArrayCoordinates
- 2D array texture coordinates
-
using Flags = Containers::
EnumSet<Flag> new in 2020.06 - Flags.
Public static functions
- static auto compile(const Configuration& configuration = Configuration{}) -> CompileState new in Git master
- Compile asynchronously.
- static auto compile(Flags flags) -> CompileState deprecated in Git master
- Compile asynchronously.
- static auto compile(Flags flags, UnsignedInt materialCount, UnsignedInt drawCount) -> CompileState deprecated in Git master
- Compile for a multi-draw scenario asynchronously.
Constructors, destructors, conversion operators
- DistanceFieldVectorGL(const Configuration& configuration = Configuration{}) explicit new in Git master
- Constructor.
- DistanceFieldVectorGL(Flags flags) deprecated in Git master explicit
- Constructor.
- DistanceFieldVectorGL(Flags flags, UnsignedInt materialCount, UnsignedInt drawCount) deprecated in Git master explicit
- Construct for a multi-draw scenario.
- DistanceFieldVectorGL(CompileState&& state) explicit new in Git master
- Finalize an asynchronous compilation.
- DistanceFieldVectorGL(NoCreateT) explicit noexcept
- Construct without creating the underlying OpenGL object.
- DistanceFieldVectorGL(const DistanceFieldVectorGL<dimensions>&) deleted
- Copying is not allowed.
- DistanceFieldVectorGL(DistanceFieldVectorGL<dimensions>&&) defaulted noexcept
- Move constructor.
Public functions
- auto operator=(const DistanceFieldVectorGL<dimensions>&) -> DistanceFieldVectorGL<dimensions>& deleted
- Copying is not allowed.
- auto operator=(DistanceFieldVectorGL<dimensions>&&) -> DistanceFieldVectorGL<dimensions>& defaulted noexcept
- Move assignment.
- auto flags() const -> Flags new in 2020.06
- Flags.
- auto materialCount() const -> UnsignedInt new in Git master
- Material count.
- auto drawCount() const -> UnsignedInt new in Git master
- Draw count.
Uniform setters
Used only if Flag::
- auto setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix) -> DistanceFieldVectorGL<dimensions>&
- Set transformation and projection matrix.
- auto setTextureMatrix(const Matrix3& matrix) -> DistanceFieldVectorGL<dimensions>& new in 2020.06
- Set texture coordinate transformation matrix.
- auto setTextureLayer(UnsignedInt layer) -> DistanceFieldVectorGL<dimensions>& new in Git master
- Set texture array layer.
- auto setColor(const Color4& color) -> DistanceFieldVectorGL<dimensions>&
- Set fill color.
- auto setOutlineColor(const Color4& color) -> DistanceFieldVectorGL<dimensions>&
- Set outline color.
- auto setOutlineRange(Float start, Float end) -> DistanceFieldVectorGL<dimensions>&
- Set outline range.
- auto setSmoothness(Float value) -> DistanceFieldVectorGL<dimensions>&
- Set smoothness radius.
Texture binding
-
auto bindVectorTexture(GL::
Texture2D& texture) -> DistanceFieldVectorGL<dimensions>& - Bind a vector texture.
-
auto bindVectorTexture(GL::
Texture2DArray& texture) -> DistanceFieldVectorGL<dimensions>& new in Git master - Bind a vector array texture.
Enum documentation
template<UnsignedInt dimensions>
enum Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: (anonymous): UnsignedInt
Enumerators | |
---|---|
ColorOutput |
Color shader output. Generic output, present always. Expects three- or four-component floating-point or normalized buffer attachment. |
template<UnsignedInt dimensions>
enum class Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: Flag: UnsignedByte new in 2020.06
Flag.
Enumerators | |
---|---|
TextureTransformation new in 2020.06 |
Enable texture coordinate transformation. |
UniformBuffers new in Git master |
Use uniform buffers. Expects that uniform data are supplied via bindTransformationProjectionBuffer(), bindDrawBuffer(), bindTextureTransformationBuffer(), and bindMaterialBuffer() instead of direct uniform setters. |
ShaderStorageBuffers new in Git master |
Use shader storage buffers. Superset of functionality provided by Flag:: |
MultiDraw new in Git master |
Enable multidraw functionality. Implies Flag:: |
TextureArrays new in Git master |
Use 2D texture arrays. Expects that the texture is supplied via bindVectorTexture(GL:: |
Typedef documentation
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::Position Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: Position
Vertex position.
Generic attribute, Vector2 in 2D, Vector3 in 3D.
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::TextureCoordinates Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: TextureCoordinates
2D texture coordinates
Generic attribute, Vector2. Use either this or the TextureArrayCoordinates attribute.
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::TextureArrayCoordinates Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: TextureArrayCoordinates
2D array texture coordinates
Generic attribute, Vector3. Use either this or the TextureCoordinates attribute. The third component is used only if Flag::
template<UnsignedInt dimensions>
typedef Containers:: EnumSet<Flag> Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: Flags new in 2020.06
Flags.
Function documentation
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: compile(const Configuration& configuration = Configuration{}) new in Git master
Compile asynchronously.
Compared to DistanceFieldVectorGL(const Configuration&) can perform an asynchronous compilation and linking. See Async shader compilation and linking for more information.
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: compile(Flags flags)
Compile asynchronously.
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: compile(Flags flags,
UnsignedInt materialCount,
UnsignedInt drawCount)
Compile for a multi-draw scenario asynchronously.
template<UnsignedInt dimensions>
Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: DistanceFieldVectorGL(Flags flags) explicit
Constructor.
template<UnsignedInt dimensions>
Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: DistanceFieldVectorGL(Flags flags,
UnsignedInt materialCount,
UnsignedInt drawCount) explicit
Construct for a multi-draw scenario.
template<UnsignedInt dimensions>
Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: DistanceFieldVectorGL(CompileState&& state) explicit new in Git master
Finalize an asynchronous compilation.
Takes an asynchronous compilation state returned by compile() and forms a ready-to-use shader object. See Async shader compilation and linking for more information.
template<UnsignedInt dimensions>
Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: DistanceFieldVectorGL(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to a moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active. However note that this is a low-level and a potentially dangerous API, see the documentation of NoCreate for alternatives.
template<UnsignedInt dimensions>
Flags Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: flags() const new in 2020.06
Flags.
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: materialCount() const new in Git master
Material count.
Statically defined size of the DistanceFieldVectorMaterialUniform uniform buffer bound with bindMaterialBuffer(). Has use only if Flag::
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: drawCount() const new in Git master
Draw count.
Statically defined size of each of the TransformationProjectionUniform2D / TransformationProjectionUniform3D, DistanceFieldVectorDrawUniform and TextureTransformationUniform uniform buffers bound with bindTransformationProjectionBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer(). Has use only if Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix)
Set transformation and projection matrix.
Returns | Reference to self (for method chaining) |
---|
Initial value is an identity matrix.
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setTextureMatrix(const Matrix3& matrix) new in 2020.06
Set texture coordinate transformation matrix.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setTextureLayer(UnsignedInt layer) new in Git master
Set texture array layer.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0
. If a three-component TextureArrayCoordinates attribute is used instead of TextureCoordinates, this value is added to the layer coming from the third component.
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setColor(const Color4& color)
Set fill color.
Returns | Reference to self (for method chaining) |
---|
Initial value is 0xffffffff_rgbaf
.
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setOutlineColor(const Color4& color)
Set outline color.
Returns | Reference to self (for method chaining) |
---|
Initial value is 0x00000000_rgbaf
and the outline is not drawn — see setOutlineRange() for more information.
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setOutlineRange(Float start,
Float end)
Set outline range.
Returns | Reference to self (for method chaining) |
---|
The start
parameter describes where fill ends and possible outline starts. Initial value is 0.5f
, larger values will make the vector art look thinner, smaller will make it look thicker.
The end
parameter describes where outline ends. If set to a value larger than start
, the outline is not drawn. Initial value is 1.0f
.
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setSmoothness(Float value)
Set smoothness radius.
Returns | Reference to self (for method chaining) |
---|
Larger values will make edges look less aliased (but blurry), smaller values will make them look more crisp (but possibly aliased). Initial value is 0.04f
.
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: setDrawOffset(UnsignedInt offset) new in Git master
Set a draw offset.
Returns | Reference to self (for method chaining) |
---|
Specifies which item in the TransformationProjectionUniform2D / TransformationProjectionUniform3D, DistanceFieldVectorDrawUniform and TextureTransformationUniform buffers bound with bindTransformationProjectionBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer() should be used for current draw. Expects that Flag::offset
is less than drawCount(). Initial value is 0
, if drawCount() is 1
, the function is a no-op as the shader assumes draw offset to be always zero.
If Flag::gl_DrawID
is added to this value, which makes each draw submitted via GL::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindTransformationProjectionBuffer(GL:: Buffer& buffer) new in Git master
Bind a transformation and projection uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindTransformationProjectionBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindDrawBuffer(GL:: Buffer& buffer) new in Git master
Bind a draw uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindDrawBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindTextureTransformationBuffer(GL:: Buffer& buffer) new in Git master
Bind a texture transformation uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that both Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindTextureTransformationBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindMaterialBuffer(GL:: Buffer& buffer) new in Git master
Bind a material uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindMaterialBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindVectorTexture(GL:: Texture2D& texture)
Bind a vector texture.
Returns | Reference to self (for method chaining) |
---|
If Flag::
template<UnsignedInt dimensions>
DistanceFieldVectorGL<dimensions>& Magnum:: Shaders:: DistanceFieldVectorGL<dimensions>:: bindVectorTexture(GL:: Texture2DArray& texture) new in Git master
Bind a vector array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0
is picked. Additionally, if Flag::
template<UnsignedInt dimensions>
template<UnsignedInt dimensions>
Debug& operator<<(Debug& debug,
DistanceFieldVectorGL<dimensions>::Flag value)
Debug output operator.
template<UnsignedInt dimensions>
template<UnsignedInt dimensions>
Debug& operator<<(Debug& debug,
DistanceFieldVectorGL<dimensions>::Flags value)
Debug output operator.