#include <Magnum/Shaders/FlatGL.h>
template<UnsignedInt dimensions>
FlatGL class new in Git master
Flat OpenGL shader.
Draws the whole mesh with given color or texture. For a colored mesh you need to provide the Position attribute in your triangle mesh. By default, the shader renders the mesh with a white color in an identity transformation. Use setTransformationProjectionMatrix(), setColor() and others to configure the shader.
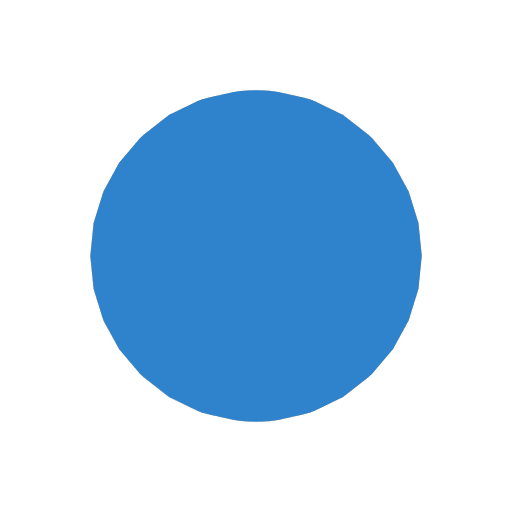
Colored rendering
Common mesh setup:
struct Vertex { Vector3 position; }; Vertex data[60]{ //... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::FlatGL3D::Position{}) // ... ;
Common rendering setup:
Matrix4 transformationMatrix = Matrix4::translation(Vector3::zAxis(-5.0f)); Matrix4 projectionMatrix = Matrix4::perspectiveProjection(35.0_degf, 1.0f, 0.001f, 100.0f); Shaders::FlatGL3D shader; shader.setColor(0x2f83cc_rgbf) .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) .draw(mesh);
Textured rendering
If you want to use a texture, you need to provide also the TextureCoordinates attribute. Pass Flag::0xffffffff_rgbaf
. Common mesh setup:
struct Vertex { Vector3 position; Vector2 textureCoordinates; }; Vertex data[60]{ // ... }; GL::Buffer vertices; vertices.setData(data, GL::BufferUsage::StaticDraw); GL::Mesh mesh; mesh.addVertexBuffer(vertices, 0, Shaders::FlatGL3D::Position{}, Shaders::FlatGL3D::TextureCoordinates{}) // ... ;
Common rendering setup:
Matrix4 transformationMatrix, projectionMatrix; GL::Texture2D texture; Shaders::FlatGL3D shader{Shaders::FlatGL3D::Configuration{} .setFlags(Shaders::FlatGL3D::Flag::Textured)}; shader.setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) .bindTexture(texture) .draw(mesh);
For coloring the texture based on intensity you can use the VectorGL shader. The 3D version of this shader is equivalent to PhongGL with zero lights, however this implementation is much simpler and thus likely also faster. See its documentation for more information. Conversely, enabling Flag::
Alpha blending and masking
Alpha / transparency is supported by the shader implicitly, but to have it working on the framebuffer, you need to enable GL::
An alternative is to enable Flag::discard
operation which is known to have considerable performance impact on some platforms. With proper depth sorting and blending you'll usually get much better performance and output quality.
Object ID output
The shader supports writing object ID to the framebuffer for object picking or other annotation purposes. Enable it using Flag::
GL::Renderbuffer color, objectId; color.setStorage(GL::RenderbufferFormat::RGBA8, size); objectId.setStorage(GL::RenderbufferFormat::R16UI, size); // large as needed framebuffer.attachRenderbuffer(GL::Framebuffer::ColorAttachment{0}, color) .attachRenderbuffer(GL::Framebuffer::ColorAttachment{1}, objectId); Shaders::FlatGL3D shader{Shaders::FlatGL3D::Configuration{} .setFlags(Shaders::FlatGL3D::Flag::ObjectId)}; // ... framebuffer.mapForDraw({ {Shaders::FlatGL3D::ColorOutput, GL::Framebuffer::ColorAttachment{0}}, {Shaders::FlatGL3D::ObjectIdOutput, GL::Framebuffer::ColorAttachment{1}}}) .clearColor(0, 0x1f1f1f_rgbf) .clearColor(1, Vector4ui{0}) .bind(); shader.setObjectId(meshId) .draw(mesh);
If you have a batch of meshes with different object IDs, enable Flag::
Skinning
To render skinned meshes, bind up to two sets of up to four-component joint ID and weight attributes to JointIds / SecondaryJointIds and Weights / SecondaryWeights, set an appropriate joint count and per-vertex primary and secondary joint count in Configuration::
To avoid having to compile multiple shader variants for different joint matrix counts, set the maximum used joint count in Configuration::
Instanced rendering
Enabling Flag::
struct { Matrix4 transformation; Color3 color; } instanceData[] { {Matrix4::translation({1.0f, 2.0f, 0.0f}), 0xff3333_rgbf}, {Matrix4::translation({2.0f, 1.0f, 0.0f}), 0x33ff33_rgbf}, {Matrix4::translation({3.0f, 0.0f, 1.0f}), 0x3333ff_rgbf}, // ... }; mesh.setInstanceCount(Containers::arraySize(instanceData)) .addVertexBufferInstanced(GL::Buffer{instanceData}, 1, 0, Shaders::FlatGL3D::TransformationMatrix{}, Shaders::FlatGL3D::Color3{});
For instanced skinning the joint buffer is assumed to contain joint transformations for all instances. By default all instances use the same joint transformations, seting setPerInstanceJointCount() will cause the shader to offset the per-vertex joint IDs with gl_InstanceID*perInstanceJointCount
.
Uniform buffers
See Using uniform buffers for a high-level overview that applies to all shaders. In this particular case, because the shader doesn't need a separate projection and transformation matrix, a combined one is supplied via a TransformationProjectionUniform2D / TransformationProjectionUniform3D buffer bound with bindTransformationProjectionBuffer(). To maximize use of the limited uniform buffer memory, materials are supplied separately in a FlatMaterialUniform buffer bound with bindMaterialBuffer() and then referenced via materialId from a FlatDrawUniform bound with bindDrawBuffer(); for optional texture transformation a per-draw TextureTransformationUniform buffer bound with bindTextureTransformationBuffer() can be supplied as well. A uniform buffer setup equivalent to the colored case at the top would look like this:
GL::Buffer transformationProjectionUniform, materialUniform, drawUniform; transformationProjectionUniform.setData({ Shaders::TransformationProjectionUniform3D{} .setTransformationProjectionMatrix(projectionMatrix*transformationMatrix) }); materialUniform.setData({ Shaders::FlatMaterialUniform{} .setColor(0x2f83cc_rgbf) }); drawUniform.setData({ Shaders::FlatDrawUniform{} .setMaterialId(0) }); Shaders::FlatGL3D shader{Shaders::FlatGL3D::Configuration{} .setFlags(Shaders::FlatGL3D::Flag::UniformBuffers)}; shader .bindTransformationProjectionBuffer(transformationProjectionUniform) .bindMaterialBuffer(materialUniform) .bindDrawBuffer(drawUniform) .draw(mesh);
For a multidraw workflow enable Flag::
For skinning, joint matrices are supplied via a TransformationUniform2D / TransformationUniform3D buffer bound with bindJointBuffer(). In an instanced scenario the per-instance joint count is supplied via FlatDrawUniform::gl_InstanceID*perInstanceJointCount + jointOffset
. The setPerVertexJointCount() stays as an immediate uniform in the UBO and multidraw scenario as well, as it is tied to a particular mesh layout and thus doesn't need to vary per draw.
Base classes
- class Magnum::GL::AbstractShaderProgram
- Base for shader program implementations.
Derived classes
- class Magnum::Shaders::FlatGL::CompileState new in Git master
- Asynchronous compilation state.
Public types
- class CompileState new in Git master
- Asynchronous compilation state.
- class Configuration new in Git master
- Configuration.
- enum (anonymous): UnsignedInt { ColorOutput = GenericGL<dimensions>::ColorOutput new in 2019.10, ObjectIdOutput = GenericGL<dimensions>::ObjectIdOutput new in 2019.10 }
- enum class Flag: UnsignedShort { Textured = 1 << 0, AlphaMask = 1 << 1, VertexColor = 1 << 2 new in 2019.10, TextureTransformation = 1 << 3 new in 2020.06, ObjectId = 1 << 4 new in 2019.10, InstancedObjectId = (1 << 5)|ObjectId new in 2020.06, ObjectIdTexture = (1 << 11)|ObjectId new in Git master, InstancedTransformation = 1 << 6 new in 2020.06, InstancedTextureOffset = (1 << 7)|TextureTransformation new in 2020.06, UniformBuffers = 1 << 8 new in Git master, ShaderStorageBuffers = UniformBuffers|(1 << 13) new in Git master, MultiDraw = UniformBuffers|(1 << 9) new in Git master, TextureArrays = 1 << 10 new in Git master, DynamicPerVertexJointCount = 1 << 12 new in Git master }
- Flag.
- using Position = GenericGL<dimensions>::Position
- Vertex position.
- using TextureCoordinates = GenericGL<dimensions>::TextureCoordinates
- 2D texture coordinates
- using Color3 = GenericGL<dimensions>::Color3 new in 2019.10
- Three-component vertex color.
- using Color4 = GenericGL<dimensions>::Color4 new in 2019.10
- Four-component vertex color.
-
using JointIds = GenericGL3D::
JointIds new in Git master - Joint ids.
-
using Weights = GenericGL3D::
Weights new in Git master - Weights.
-
using SecondaryJointIds = GenericGL3D::
SecondaryJointIds new in Git master - Secondary joint ids.
-
using SecondaryWeights = GenericGL3D::
SecondaryWeights new in Git master - Secondary weights.
- using ObjectId = GenericGL<dimensions>::ObjectId new in 2020.06
- (Instanced) object ID
- using TransformationMatrix = GenericGL<dimensions>::TransformationMatrix new in 2020.06
- (Instanced) transformation matrix
- using TextureOffset = GenericGL<dimensions>::TextureOffset new in 2020.06
- (Instanced) texture offset
- using TextureOffsetLayer = GenericGL<dimensions>::TextureOffsetLayer new in Git master
- (Instanced) texture offset and layer
-
using Flags = Containers::
EnumSet<Flag> - Flags.
Public static functions
- static auto compile(const Configuration& configuration = Configuration{}) -> CompileState new in Git master
- Compile asynchronously.
- static auto compile(Flags flags) -> CompileState deprecated in Git master
- Compile asynchronously.
- static auto compile(Flags flags, UnsignedInt materialCount, UnsignedInt drawCount) -> CompileState deprecated in Git master
- Compile for a multi-draw scenario asynchronously.
Constructors, destructors, conversion operators
- FlatGL(const Configuration& configuration = Configuration{}) explicit new in Git master
- Constructor.
- FlatGL(Flags flags) deprecated in Git master explicit
- Constructor.
- FlatGL(Flags flags, UnsignedInt materialCount, UnsignedInt drawCount) deprecated in Git master explicit
- Construct for a multi-draw scenario.
- FlatGL(CompileState&& state) explicit new in Git master
- Finalize an asynchronous compilation.
- FlatGL(NoCreateT) explicit noexcept
- Construct without creating the underlying OpenGL object.
- FlatGL(const FlatGL<dimensions>&) deleted
- Copying is not allowed.
- FlatGL(FlatGL<dimensions>&&) defaulted noexcept
- Move constructor.
Public functions
- auto operator=(const FlatGL<dimensions>&) -> FlatGL<dimensions>& deleted
- Copying is not allowed.
- auto operator=(FlatGL<dimensions>&&) -> FlatGL<dimensions>& defaulted noexcept
- Move assignment.
- auto flags() const -> Flags
- Flags.
- auto jointCount() const -> UnsignedInt new in Git master
- Joint count.
- auto perVertexJointCount() const -> UnsignedInt new in Git master
- Per-vertex joint count.
- auto secondaryPerVertexJointCount() const -> UnsignedInt new in Git master
- Secondary per-vertex joint count.
- auto materialCount() const -> UnsignedInt new in Git master
- Material count.
- auto drawCount() const -> UnsignedInt new in Git master
- Draw count.
- auto setPerVertexJointCount(UnsignedInt count, UnsignedInt secondaryCount = 0) -> FlatGL<dimensions>& new in Git master
- Set dynamic per-vertex skinning joint count.
Uniform setters
Used only if Flag::
- auto setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix) -> FlatGL<dimensions>&
- Set transformation and projection matrix.
- auto setTextureMatrix(const Matrix3& matrix) -> FlatGL<dimensions>& new in 2020.06
- Set texture coordinate transformation matrix.
- auto setTextureLayer(UnsignedInt layer) -> FlatGL<dimensions>& new in Git master
- Set texture array layer.
-
auto setColor(const Magnum::
Color4& color) -> FlatGL<dimensions>& - Set color.
- auto setAlphaMask(Float mask) -> FlatGL<dimensions>&
- Set alpha mask value.
- auto setObjectId(UnsignedInt id) -> FlatGL<dimensions>&
- Set object ID.
-
auto setJointMatrices(Containers::
ArrayView<const MatrixTypeFor<dimensions, Float>> matrices) -> FlatGL<dimensions>& new in Git master - Set joint matrices.
-
auto setJointMatrices(std::
initializer_list<MatrixTypeFor<dimensions, Float>> matrices) -> FlatGL<dimensions>& new in Git master - auto setJointMatrix(UnsignedInt id, const MatrixTypeFor<dimensions, Float>& matrix) -> FlatGL<dimensions>& new in Git master
- Set joint matrix for given joint.
- auto setPerInstanceJointCount(UnsignedInt count) -> FlatGL<dimensions>& new in Git master
- Set per-instance joint count.
Texture binding
-
auto bindTexture(GL::
Texture2D& texture) -> FlatGL<dimensions>& - Bind a color texture.
-
auto bindTexture(GL::
Texture2DArray& texture) -> FlatGL<dimensions>& new in Git master - Bind a color array texture.
-
auto bindObjectIdTexture(GL::
Texture2D& texture) -> FlatGL<dimensions>& new in Git master - Bind an object ID texture.
-
auto bindObjectIdTexture(GL::
Texture2DArray& texture) -> FlatGL<dimensions>& new in Git master - Bind an object ID array texture.
Enum documentation
template<UnsignedInt dimensions>
enum Magnum:: Shaders:: FlatGL<dimensions>:: (anonymous): UnsignedInt
Enumerators | |
---|---|
ColorOutput new in 2019.10 |
Color shader output. Generic output, present always. Expects three- or four-component floating-point or normalized buffer attachment. |
ObjectIdOutput new in 2019.10 |
Object ID shader output. Generic output, present only if Flag:: |
template<UnsignedInt dimensions>
enum class Magnum:: Shaders:: FlatGL<dimensions>:: Flag: UnsignedShort
Flag.
Enumerators | |
---|---|
Textured |
Multiply color with a texture. |
AlphaMask |
Enable alpha masking. If the combined fragment color has an alpha less than the value specified with setAlphaMask(), given fragment is discarded. This uses the |
VertexColor new in 2019.10 |
Multiply the color with a vertex color. Requires either the Color3 or Color4 attribute to be present. |
TextureTransformation new in 2020.06 |
Enable texture coordinate transformation. If this flag is set, the shader expects that Flag:: |
ObjectId new in 2019.10 |
Enable object ID output. See Object ID output for more information. |
InstancedObjectId new in 2020.06 |
Instanced object ID. Retrieves a per-instance / per-vertex object ID from the ObjectId attribute, outputting a sum of the per-vertex ID and ID coming from setObjectId() or FlatDrawUniform:: |
ObjectIdTexture new in Git master |
Object ID texture. Retrieves object IDs from a texture bound with bindObjectIdTexture(), outputting a sum of the object ID texture, the ID coming from setObjectId() or FlatDrawUniform:: |
InstancedTransformation new in 2020.06 |
Instanced transformation. Retrieves a per-instance transformation matrix from the TransformationMatrix attribute and uses it together with the matrix coming from setTransformationProjectionMatrix() or TransformationProjectionUniform2D:: |
InstancedTextureOffset new in 2020.06 |
Instanced texture offset. Retrieves a per-instance offset vector from the TextureOffset attribute and uses it together with the matrix coming from setTextureMatrix() or TextureTransformationUniform:: If Flag:: |
UniformBuffers new in Git master |
Use uniform buffers. Expects that uniform data are supplied via bindTransformationProjectionBuffer(), bindDrawBuffer(), bindTextureTransformationBuffer() and bindMaterialBuffer() instead of direct uniform setters. |
ShaderStorageBuffers new in Git master |
Use shader storage buffers. Superset of functionality provided by Flag:: |
MultiDraw new in Git master |
Enable multidraw functionality. Implies Flag:: |
TextureArrays new in Git master |
Use 2D texture arrays. Expects that the texture is supplied via bindTexture(GL:: |
DynamicPerVertexJointCount new in Git master |
Dynamic per-vertex joint count for skinning. Uses only the first M / N primary / secondary components defined by setPerVertexJointCount() instead of all primary / secondary components defined by Configuration:: |
Typedef documentation
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::Position Magnum:: Shaders:: FlatGL<dimensions>:: Position
Vertex position.
Generic attribute, Vector2 in 2D, Vector3 in 3D.
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::TextureCoordinates Magnum:: Shaders:: FlatGL<dimensions>:: TextureCoordinates
2D texture coordinates
Generic attribute, Vector2. Used only if Flag::
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::Color3 Magnum:: Shaders:: FlatGL<dimensions>:: Color3 new in 2019.10
Three-component vertex color.
Generic attribute, Magnum::
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::Color4 Magnum:: Shaders:: FlatGL<dimensions>:: Color4 new in 2019.10
Four-component vertex color.
Generic attribute, Magnum::
template<UnsignedInt dimensions>
typedef GenericGL3D:: JointIds Magnum:: Shaders:: FlatGL<dimensions>:: JointIds new in Git master
Joint ids.
Generic attribute, Vector4ui. Used only if perVertexJointCount() isn't 0
.
template<UnsignedInt dimensions>
typedef GenericGL3D:: Weights Magnum:: Shaders:: FlatGL<dimensions>:: Weights new in Git master
Weights.
Generic attribute, Vector4. Used only if perVertexJointCount() isn't 0
.
template<UnsignedInt dimensions>
typedef GenericGL3D:: SecondaryJointIds Magnum:: Shaders:: FlatGL<dimensions>:: SecondaryJointIds new in Git master
Secondary joint ids.
Generic attribute, Vector4ui. Used only if secondaryPerVertexJointCount() isn't 0
.
template<UnsignedInt dimensions>
typedef GenericGL3D:: SecondaryWeights Magnum:: Shaders:: FlatGL<dimensions>:: SecondaryWeights new in Git master
Secondary weights.
Generic attribute, Vector4. Used only if secondaryPerVertexJointCount() isn't 0
.
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::ObjectId Magnum:: Shaders:: FlatGL<dimensions>:: ObjectId new in 2020.06
(Instanced) object ID
Generic attribute, UnsignedInt. Used only if Flag::
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::TransformationMatrix Magnum:: Shaders:: FlatGL<dimensions>:: TransformationMatrix new in 2020.06
(Instanced) transformation matrix
Generic attribute, Matrix3 in 2D, Matrix4 in 3D. Used only if Flag::
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::TextureOffset Magnum:: Shaders:: FlatGL<dimensions>:: TextureOffset new in 2020.06
(Instanced) texture offset
Generic attribute, Vector2. Use either this or the TextureOffsetLayer attribute. Used only if Flag::
template<UnsignedInt dimensions>
typedef GenericGL<dimensions>::TextureOffsetLayer Magnum:: Shaders:: FlatGL<dimensions>:: TextureOffsetLayer new in Git master
(Instanced) texture offset and layer
Generic attribute, Vector3, with the last component interpreted as an integer. Use either this or the TextureOffset attribute. First two components used only if Flag::
template<UnsignedInt dimensions>
typedef Containers:: EnumSet<Flag> Magnum:: Shaders:: FlatGL<dimensions>:: Flags
Flags.
Function documentation
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: FlatGL<dimensions>:: compile(const Configuration& configuration = Configuration{}) new in Git master
Compile asynchronously.
Compared to FlatGL(const Configuration&) can perform an asynchronous compilation and linking. See Async shader compilation and linking for more information.
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: FlatGL<dimensions>:: compile(Flags flags)
Compile asynchronously.
template<UnsignedInt dimensions>
static CompileState Magnum:: Shaders:: FlatGL<dimensions>:: compile(Flags flags,
UnsignedInt materialCount,
UnsignedInt drawCount)
Compile for a multi-draw scenario asynchronously.
template<UnsignedInt dimensions>
Magnum:: Shaders:: FlatGL<dimensions>:: FlatGL(Flags flags) explicit
Constructor.
template<UnsignedInt dimensions>
Magnum:: Shaders:: FlatGL<dimensions>:: FlatGL(Flags flags,
UnsignedInt materialCount,
UnsignedInt drawCount) explicit
Construct for a multi-draw scenario.
template<UnsignedInt dimensions>
Magnum:: Shaders:: FlatGL<dimensions>:: FlatGL(CompileState&& state) explicit new in Git master
Finalize an asynchronous compilation.
Takes an asynchronous compilation state returned by compile() and forms a ready-to-use shader object. See Async shader compilation and linking for more information.
template<UnsignedInt dimensions>
Magnum:: Shaders:: FlatGL<dimensions>:: FlatGL(NoCreateT) explicit noexcept
Construct without creating the underlying OpenGL object.
The constructed instance is equivalent to a moved-from state. Useful in cases where you will overwrite the instance later anyway. Move another object over it to make it useful.
This function can be safely used for constructing (and later destructing) objects even without any OpenGL context being active. However note that this is a low-level and a potentially dangerous API, see the documentation of NoCreate for alternatives.
template<UnsignedInt dimensions>
Flags Magnum:: Shaders:: FlatGL<dimensions>:: flags() const
Flags.
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: FlatGL<dimensions>:: jointCount() const new in Git master
Joint count.
If Flag::
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: FlatGL<dimensions>:: perVertexJointCount() const new in Git master
Per-vertex joint count.
Returns the value set with Configuration::
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: FlatGL<dimensions>:: secondaryPerVertexJointCount() const new in Git master
Secondary per-vertex joint count.
Returns the value set with Configuration::
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: FlatGL<dimensions>:: materialCount() const new in Git master
Material count.
Statically defined size of the FlatMaterialUniform uniform buffer bound with bindMaterialBuffer(). Has use only if Flag::
template<UnsignedInt dimensions>
UnsignedInt Magnum:: Shaders:: FlatGL<dimensions>:: drawCount() const new in Git master
Draw count.
Statically defined size of each of the TransformationProjectionUniform2D / TransformationProjectionUniform3D, FlatDrawUniform and TextureTransformationUniform uniform buffers bound with bindTransformationProjectionBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer(). Has use only if Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setPerVertexJointCount(UnsignedInt count,
UnsignedInt secondaryCount = 0) new in Git master
Set dynamic per-vertex skinning joint count.
Returns | Reference to self (for method chaining) |
---|
Allows reducing the count of iterated joints for a particular draw call, making it possible to use a single shader with meshes that contain different count of per-vertex joints. See Flag::
Expects that Flag::count
is not larger than perVertexJointCount() and secondaryCount
not larger than secondaryPerVertexJointCount().
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setTransformationProjectionMatrix(const MatrixTypeFor<dimensions, Float>& matrix)
Set transformation and projection matrix.
Returns | Reference to self (for method chaining) |
---|
Initial value is an identity matrix. If Flag::
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setTextureMatrix(const Matrix3& matrix) new in 2020.06
Set texture coordinate transformation matrix.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setTextureLayer(UnsignedInt layer) new in Git master
Set texture array layer.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0
. If Flag::
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setColor(const Magnum:: Color4& color)
Set color.
Returns | Reference to self (for method chaining) |
---|
Initial value is 0xffffffff_rgbaf
. If Flag::
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setAlphaMask(Float mask)
Set alpha mask value.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0.5f
. See the flag documentation for further information.
This corresponds to glAlphaFunc() in classic OpenGL.
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setObjectId(UnsignedInt id)
Set object ID.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::0
. If Flag::
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setJointMatrices(Containers:: ArrayView<const MatrixTypeFor<dimensions, Float>> matrices) new in Git master
Set joint matrices.
Returns | Reference to self (for method chaining) |
---|
Initial values are identity transformations. Expects that the size of the matrices
array is not larger than jointCount().
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setJointMatrices(std:: initializer_list<MatrixTypeFor<dimensions, Float>> matrices) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setJointMatrix(UnsignedInt id,
const MatrixTypeFor<dimensions, Float>& matrix) new in Git master
Set joint matrix for given joint.
Returns | Reference to self (for method chaining) |
---|
Unlike setJointMatrices() updates just a single joint matrix. Expects that id
is less than jointCount().
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setPerInstanceJointCount(UnsignedInt count) new in Git master
Set per-instance joint count.
Returns | Reference to self (for method chaining) |
---|
Offset added to joint IDs in the JointIds and SecondaryJointIds in instanced draws. Should be less than jointCount(). Initial value is 0
, meaning every instance will use the same joint matrices, setting it to a non-zero value causes the joint IDs to be interpreted as gl_InstanceID*count + jointId
.
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: setDrawOffset(UnsignedInt offset) new in Git master
Set a draw offset.
Returns | Reference to self (for method chaining) |
---|
Specifies which item in the TransformationProjectionUniform2D / TransformationProjectionUniform3D, FlatDrawUniform and TextureTransformationUniform buffers bound with bindTransformationProjectionBuffer(), bindDrawBuffer() and bindTextureTransformationBuffer() should be used for current draw. Expects that Flag::offset
is less than drawCount(). Initial value is 0
, if drawCount() is 1
, the function is a no-op as the shader assumes draw offset to be always zero.
If Flag::gl_DrawID
is added to this value, which makes each draw submitted via GL::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindTransformationProjectionBuffer(GL:: Buffer& buffer) new in Git master
Bind a transformation and projection uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindTransformationProjectionBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindDrawBuffer(GL:: Buffer& buffer) new in Git master
Bind a draw uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindDrawBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindTextureTransformationBuffer(GL:: Buffer& buffer) new in Git master
Bind a texture transformation uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that both Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindTextureTransformationBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindMaterialBuffer(GL:: Buffer& buffer) new in Git master
Bind a material uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindMaterialBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindJointBuffer(GL:: Buffer& buffer) new in Git master
Bind a joint matrix uniform / shader storage buffer.
Returns | Reference to self (for method chaining) |
---|
Expects that Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindJointBuffer(GL:: Buffer& buffer,
GLintptr offset,
GLsizeiptr size) new in Git master
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindTexture(GL:: Texture2D& texture)
Bind a color texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindTexture(GL:: Texture2DArray& texture) new in Git master
Bind a color array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with both Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindObjectIdTexture(GL:: Texture2D& texture) new in Git master
Bind an object ID texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with Flag::
template<UnsignedInt dimensions>
FlatGL<dimensions>& Magnum:: Shaders:: FlatGL<dimensions>:: bindObjectIdTexture(GL:: Texture2DArray& texture) new in Git master
Bind an object ID array texture.
Returns | Reference to self (for method chaining) |
---|
Expects that the shader was created with both Flag::
template<UnsignedInt dimensions>
template<UnsignedInt dimensions>
Debug& operator<<(Debug& debug,
FlatGL<dimensions>::Flag value)
Debug output operator.
template<UnsignedInt dimensions>
template<UnsignedInt dimensions>
Debug& operator<<(Debug& debug,
FlatGL<dimensions>::Flags value)
Debug output operator.